Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial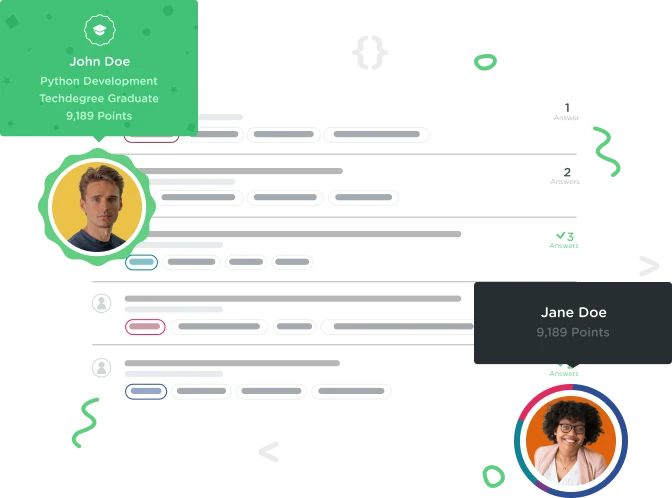

Teresa von Bitner
Courses Plus Student 1,127 PointsHow do you structure the do while loop for the battery exercise?
How do you structure the do while loop for the battery exercise?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
if (!isFullyCharged()) {
mBarsCount++;
//mBarsCount=MAX_ENERGY_BARS;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
1 Answer

Felipe Laso-Marsetti
3,158 PointsHere is the solution to the exercise:
public void charge() {
while (!isFullyCharged()) {
mBarsCount++;
}
}
Let's walk through it. The exercise says to use your new isFullyCharged method along with the ! sign using a while loop.
So what we do here is while not (!) fully charged, or in code this is the line that reads: while (!isFullyCharged), then we get inside the loop and increment mBarsCount by one using the post-increment operator (the ++ after mBarsCount).
In this case you could use either the pre-increment operator (++mBarsCount) or the post increment. The difference is that the pre-increment operator first increments the value and then uses it in any expression, where as post-increment increments the value after any expression is evaluated. Because the increment is in its own line both yield the same results and it's as if you had done mBarsCount += 1; or mBarsCount = mBarsCount + 1;
Hope this helps, and feel free to tag me in the post or ping me via Twitter (see my profile) if you need any more help with this :D
Great job on working through the exercises and keep up the effort.