Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial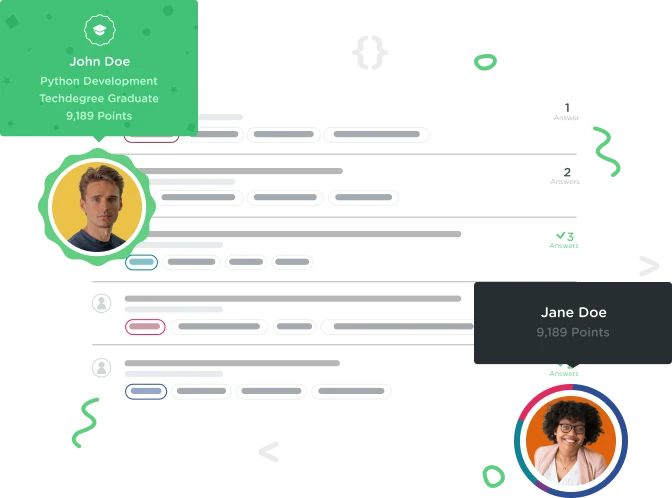
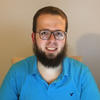
Micah Doulos
17,663 PointsHow do you target multiple elements within a list item?
We've learned how to interact with the textContent of any random list item by referencing the parent div and using event.target. But what if the list item contains multiple elements, not just text?
For example. I have a list item which contains a photo, an H3, and a table. If I click on any item in the list item, I am triggering a click event for the list item. I want to make the photo BIGGER and the H3 smaller when the list item is clicked. How is this done?
I assume something like: event.target.img.style.width="100px";
and
event.target.h3.style.fontSize=".5em";
is this correct? If not, how do you target these elements and change their style? How can you use img:nth-of-type(2) in an event.target.....style declaration?
3 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsYou could also use the Element.querySelector() method:
var target = event.target;
var heading3 = target.querySelector('h3');
var secondImage = target.querySelector('img:nth-of-type(2)');
heading3.style.fontSize = '.5em';
secondImage.style.width = '100px';

Wanderson Macêdo
9,266 PointsHi Micah, how you doing? Let me explain some things. (firstly, sorry, my English isn't good)
The target property of the event object, already is the element that trigger the event, so, it doesn't have another property called h3 or img. You need to do something else to do what you want to. Look to the code example below:
list.addEventListener('click', function(event){
target = event.target;
childrenElements = target.children;
for(let i = 0; i < childrenElements.length; i++){
if(childrenElements[i].tagName == "H3"){ childrenElements[i].style.fontSize = '.5em'; }
if(childrenElements[i].tagName == "IMG"){ childrenElements[i].style.width = '100px'; }
}
});
So, in this example, I'm getting the element that triggers the event, after that I'm getting all the elements that are children of the target, looping trough out all the elements and testing which one of them is a h3 or a Img element and then, I'm applying the style I want to. Is it clear to understand?
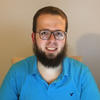
Micah Doulos
17,663 PointsThank you for your response. I see how you select by tagName, but what if there is more than one IMG and they need to be styled separately? How do you select in your for loop the 2nd IMG?

KAREN ROSS
3,026 PointsWould it be along the lines of:-
const img = childrenElements.getElementsByTagName("IMG"); img = childrenElements[i][1]
(select 2nd image)
Micah Doulos
17,663 PointsMicah Doulos
17,663 PointsI'm getting an uncaught type error: Cannot read property 'target' of undefined
Iain Simmons
Treehouse Moderator 32,305 PointsIain Simmons
Treehouse Moderator 32,305 PointsThe code above should be in a function with the
event
parameter, passed as the second argument to theaddEventListener
method, similar to the start Wanderson Macêdo's code below:list.addEventListener('click', function(event){