Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial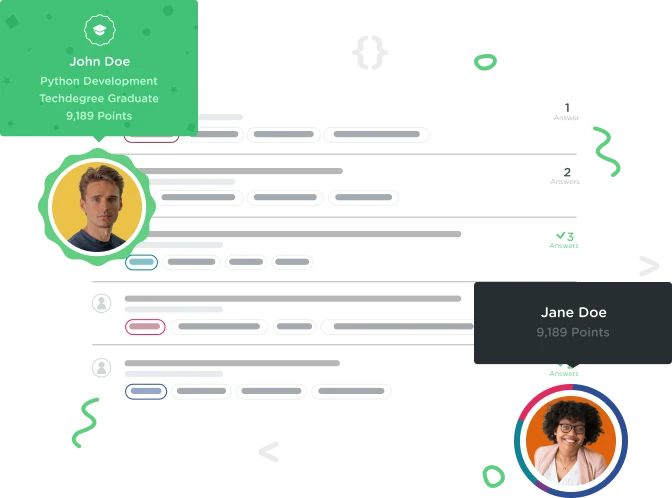

Luke Strama
6,928 PointsHow do you throw the exception before changing fields? Keep getting an error message on GoKart exceptions exercise
I believe that my code is correct here (it looks near identical to the exercise we had done just before) but I can't get it to execute properly. I've tried everything I can think of...
"Make sure you throw the exception before you change the barCount and lapsDriven fields."
Thanks!
class GoKart {
public static final int MAX_BARS = 8;
private String color;
private int barCount;
private int lapsDriven;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
public void charge() {
barCount = MAX_BARS;
}
public boolean isBatteryEmpty() {
return barCount == 0;
}
public boolean isFullyCharged() {
return MAX_BARS == barCount;
}
public void drive(int laps) {
int newLapsDriven = lapsDriven += laps;
int newBarCount = barCount -= laps;
if (newBarCount < 0) {
throw new IllegalArgumentException("Not enough juice!");
}
lapsDriven = newLapsDriven;
barCount = newBarCount;
}
public void drive() {
drive(1);
}
}
1 Answer

andren
28,558 PointsThe problem is that you use the += and -= operator in your first two lines. Those operators takes the variable on the left and adds/subtracts the value on the right to it. If your goal is just to add or subtract the values together without modifying the variable involved in the computation then you should just use the normal + and - operator.
Like this:
public void drive(int laps) {
int newLapsDriven = lapsDriven + laps; // += replaced with +
int newBarCount = barCount - laps; // -= replaced with -
if (newBarCount < 0) {
throw new IllegalArgumentException("Not enough juice!");
}
lapsDriven = newLapsDriven;
barCount = newBarCount;
}
It's worth mentining though that while that will work you didn't really need to create the newLapsDriven
and newBarCount
variables in the first place to solve this challenge. If you use laps > barCount
as the condition for your if
statement then you could have kept the original code that was in the drive
method intact.
Like this:
public void drive(int laps) {
if (laps > barCount) {
throw new IllegalArgumentException("Not enough juice!");
}
lapsDriven += laps;
barCount -= laps;
}
Luke Strama
6,928 PointsLuke Strama
6,928 PointsPerfect, that makes a lot of sense! Thanks!