Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial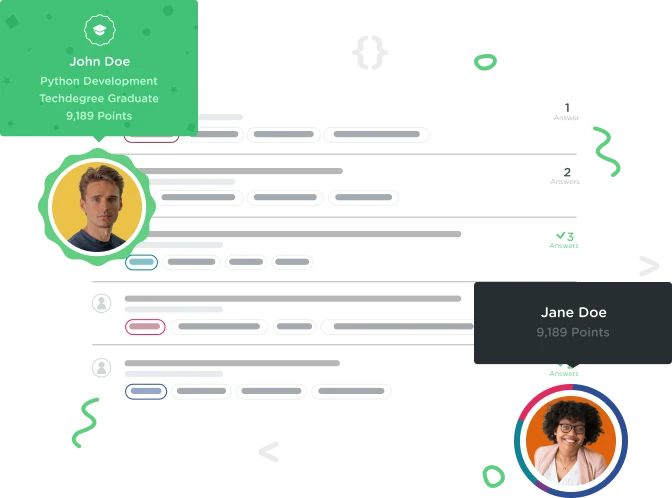

Simon Riemertzon
862 PointsHow do you work around constructors in Kotlin?
I'm trying to follow this Java tutorial here on treehouse. Its the one where you build an interactive story app. I'm trying to follow along and convert it to Kotlin code as I go. But I got into a problem. I'm trying to work out how to use constructors in Kotlin.
Constructors in Java is full-fledged methods (I gotten this explained to me by @Michael Hulet here on treehouse.) They can run any executable code you put in there at the time of initializing.
But in Kotlin, you dont need a constructor in the same way. At least in my understanding of searching the web for answers. Because in Kotlin you can set up a default constructor right in the class. Like this example:
class Page (
var imageId : Int,
var textId : Int,
var choice1 : Choice? = null,
var choice2 : Choice? = null,
var isFinalPage : Boolean = false
) {
//Do code in here.
}
//Using the class:
Page(R.drawable.page4,
R.string.page4,
Choice(R.string.page4_choice1, 5),
Choice(R.string.page4_choice2, 6)),
Page(R.drawable.page5, R.string.page5, isFinalPage = true),
Page(R.drawable.page6, R.string.page6, isFinalPage = true)
This is how I did it.
The problem here though is that I cant execute other code here, as you can with Java constructors. Like for example that "isFinalPage" boolean. In another video of the tutorial it is instead SET in a SECOND constructor, where the Page-class is initialized with only 2 parameters instead of 4.
The way I'm doing it above means that I have to change the value of "isFinalPage", when I initialize the page.class with two parameters. And I have to remember to do this. For me, this is a bit counter intuitive.
What are your thoughts on this? Is it possible to do this in another way in Kotlin that I dont know of?
1 Answer

Seth Kroger
56,415 PointsKotlin uses an "init" block to run other code for the constructor:
class Page (
// ...
) {
init {
// Do other constructor stuff here
}
}