Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial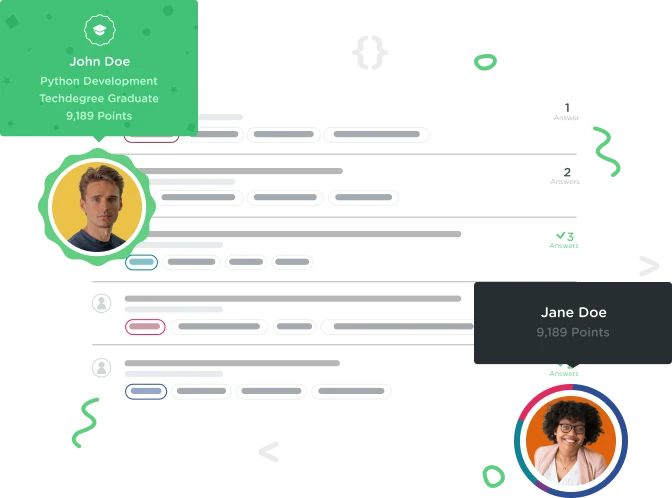
Jeff Ward
8,978 PointsHow do you 'write' this code? It seems all of the functions rely on other functions. How/Where do you start?
I am pretty new to Object Oriented Programming (OOP) to this degree. I consider myself pretty competent in JavaScript and jQuery, and now I am learning true OOP programming as seen in this project and the 'ToDo' App taught by the other instructor.
It's probably because I am a beginner and haven't had my 'Ah ha!' moment yet in regards to this:
How would one go about writing code similar to this quiz application? It seems like all of the functions rely on other code to run and execute. I am looking at the code and I can't imagine where the code 'starts' and where it ends, its all active and all the code relies on other code to run.
It seems very difficult to sit down and write this code. I imagine that you would think, I need this function, that function, and this handler, but this is very complex (again, I haven't quite grasped it yet).
It seems that when Andrew Chalkley was writing the code he was using varibles and functions he hadn't even written yet.
Any tips on better grasping this and 'tackling' a project from an OOP approach?
4 Answers
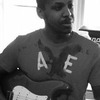
Samuel Webb
25,370 PointsJust follow the method chain (they're called methods when a function is on an object). If you're looking at a method and you notice it relies on another method, go look for that other method and see what it does. Then go back to the one you were on and you'll have a better grasp on what that does. As you read through the code, you'll start to see other methods that rely on the ones you've already read about and your understanding of the codebase will continue to grow.
To start a project like this, you go with the bare essential methods to make it work. Then you add features and refactor from there. The reason he has so many methods relying on other methods is to keep his code DRY. There's no secret method to being able to write code like this. Just practice, read other people's code and try to understand what is really happening in the code in small chunks instead of trying to know what the whole program does right from the start.
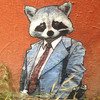
Jason Butler
5,732 PointsI'm pretty new to OOP as well, and haven't taken the Treehouse course on it, but I'd suggest starting on paper. List out what you think the needed functions might be, and some of the variables/arrays you think you'll need. Start grouping them into objects; you can also start with the broader perspective of what classes you think you'll want, and then break them down into the methods they'll hold. Draw out some UML diagrams or something similar if that helps you visualize it; draw arrows, doodles, whatever helps is fair game.
One somewhat related piece of advice I got from a good source, is to test early and test often. Write just the simplest version of a function, test it, add a little tiny piece, test, and so forth. You can use a console.log or an alert as placeholder code to see your functions working (for instance, console.log("Function executed!") as the last line of code). You'll save time tracking down errors later, and it's also satisfying to see each little piece start to add up.
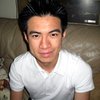
jason chan
31,009 Pointsthink of object as a way to store things.
An object can store properties, functions, and objects. Basically object is this big dictionary. That can store info and do things.
OOP javascript makes things so much easier and awesome.

Faddah Wolf
12,811 Pointspart of the beauty of the JavaScript programming language is its lauded "non-blocking, asynchronous" nature. meaning, you can send out for a data source and the rest of your code keeps running, it doesn't just do a strange halt whilst waiting for the data to return and complete; and, you can set-up, to a certain degree, your vars and functions in any order you desire, so long as they are instantiated and called properly.
thus, in JavaScript, you can have a number of functions called where you haven't read their declartion to know what they do (yet), as they are elsewhere later in your code or another *.js file you've included it in the proper order of script tags in the HTML. this is unlike PHP or other "synchronous" languages, which require you to instantiate and use your vars, functions, objects, etc., in the order they will be used/called, or you get an error. this is a double-edged sword in JavaScript, as it means you, as the developer can throw vars & functions in your code wherever you like, within reason; however, even tho' it may compile & run, too much randomness can make your code unreadable to anyone else reading/using it.
this can also get a bit mind-blowing when you get into things like "recursion" or "recursive functions," where a JavaScript function actually calls itself within that function you are currently defining. pretty weird, huh? but it works. for a good example of this, see this JavaScript recursion example video, here.
just some of the odd things to keep in mind when using JavaScript.