Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial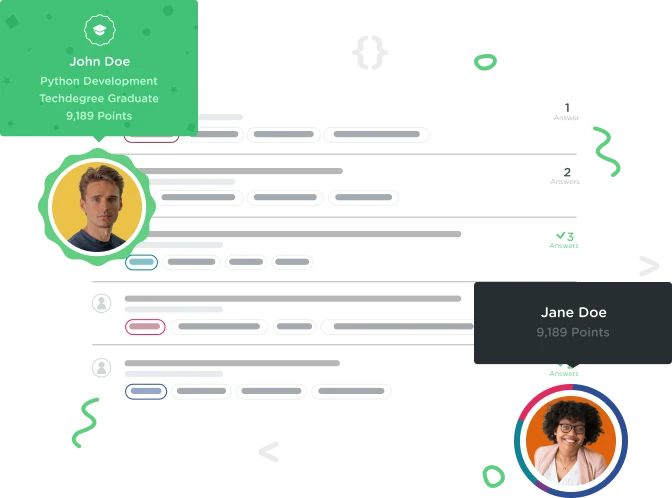

Justin Morales
5,016 PointsHow does a descendent selector work?
In my track, I have not learned about descendent selector. How does it work with the querySelectorAll() method? Does it just choose the first elements that match the given hierarchy of tags given or does choose all that match the selection? For example if I say document.querySelectorAll("section ul") , will it give me all 'ul' if it is in a section tag or will it only return all 'ul' in the first section found in the document? A bit of clarification on that would be helpful.
let navigationLinks = document.querySelectorAll('nav a');
let galleryLinks = document.querySelectorAll('section #gallery a');
let footerImages;
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>Treehouse Student | Designer</title>
<link rel="stylesheet" href="css/normalize.css">
<link href='http://fonts.googleapis.com/css?family=Changa+One|Open+Sans:400italic,700italic,400,700,800' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<link rel="stylesheet" href="css/responsive.css">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
</head>
<body>
<header>
<a href="index.html" id="logo">
<h1>Treehouse Student</h1>
<h2>Designer</h2>
</a>
<nav>
<ul>
<li><a href="#" class="selected">Work</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
</header>
<div id="wrapper">
<section>
<ul id="gallery">
<li>
<a href="#">
<img src="img/numbers-01.jpg" alt="The number 1 painted with colors and textures.">
<p>Experimentation with color and texture.</p>
</a>
</li>
<li>
<a href="#">
<img src="img/numbers-02.jpg" alt="The number 2 painted with various colors blended together.">
<p>Playing with blending modes in Photoshop.</p>
</a>
</li>
</ul>
</section>
<footer>
<a href="https://twitter.com/treehouse"><img src="img/twitter-wrap.png" alt="Twitter Logo" class="social-icon"></a>
<a href="https://www.facebook.com/TeamTreehouse"><img src="img/facebook-wrap.png" alt="Facebook Logo" class="social-icon"></a>
<p>© Treehouse Student.</p>
</footer>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
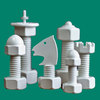
Steven Parker
231,275 PointsA descendant selector takes a space-separated list of containers followed by a target. It selects things that match the target and are also decendants (contained by) the other items. For your example of 'nav a', that selects "a" elements that are inside of a "nav" element. Note that it doesn't matter now deeply nested the elements are. For example, this would select an anchor that was a child of a nav, but it would also select one that was inside a span that was inside a div that was inside of a nav.
As you might guess from the name, the querySelectorAll method returns a list of all elements that match the selector. On the other hand, querySelector returns only the first element that matches the selector.
Your other example of querySelectorAll("section ul") would give you a list of all "ul" elements that were inside of any "selection" element.