Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial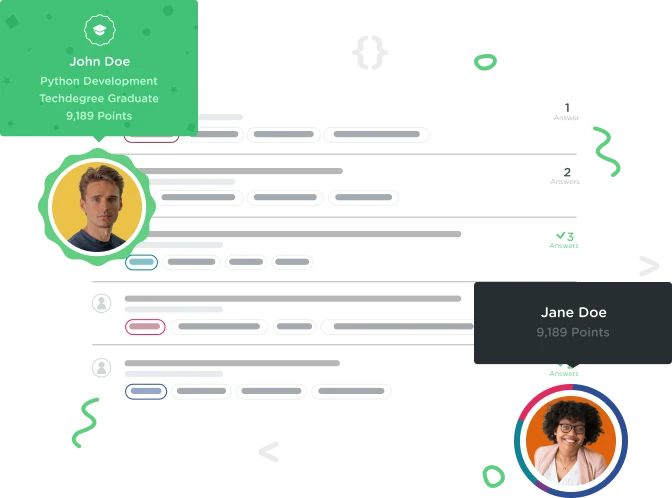
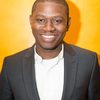
Welby Obeng
20,340 PointsHow does assertGreater and assertLess know an instance of an object is greater or lesser than the other
import unittest
import moves
class MoveTests(unittest.TestCase):
def setUp(self):
self.rock = moves.Rock()
self.paper= moves.Paper()
self.scrissors = moves.Scissors()
def test_rock_better_than_scissors(self):
self.assertGreater(self.rock, self.scrissors)
def test_paper_worse_than_scissors(
self):
self.assertLess(self.paper, self.scrissors)
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Welby,
I believe it's the __gt__
and __lt__
methods that allow the comparison to happen. You have to supply these in your class so that 'greater than' and 'less than' comparisons can be made between objects.
In general:
assertGreater(x, y)
#Checks if
x > y
# This calls
x.__gt__(y)
In the case of the code you posted:
self.assertGreater(self.rock, self.scrissors)
The __gt__
method would be called on the rock object and the scissors object would be passed in as the argument.
This is the moves.py file from the "rps" folder related to this course:
class Move:
better_than = None
worse_than = None
def __gt__(self, other):
"""Is this instance being compared to an instance from a worse class"""
return other.__class__.__name__ in self.better_than
def __lt__(self, other):
"""Is this instance being compared to an instance from a better class"""
return other.__class__.__name__ in self.worse_than
def __eq__(self, other):
"""Is this instance being compared to an instance from the same class"""
return type(other) == type(self)
def __ne__(self, other):
"""Is this instance being compared to an instance from another class"""
return other.__class__ != self.__class__
class Rock(Move):
better_than = ['Scissors']
worse_than = ['Paper']
class Paper(Move):
better_than = ['Rock']
worse_than = ['Scissors']
class Scissors(Move):
better_than = ['Paper']
worse_than = ['Rock']
Kenneth did supply both a __gt__
and __lt__
method which will return True
or False
depending on the result of the return
statement.