Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial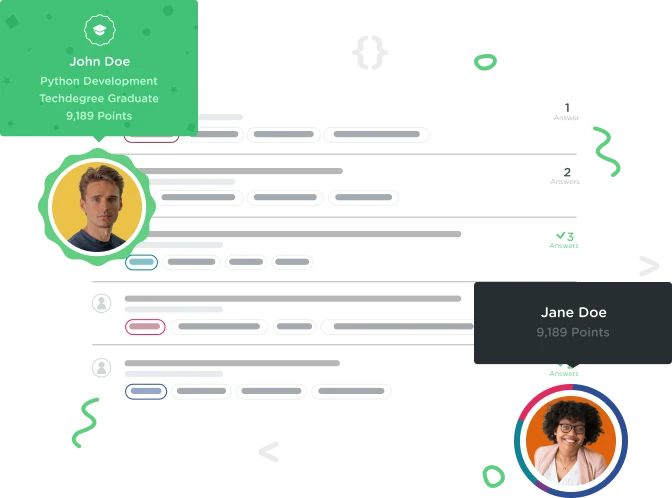

Ivan Yudhi
2,031 PointsHow does boolean work?
So I'm trying to get a better understanding of how boolean works
for the method below..
public boolean isEmpty() {
return mPezCount == 0;
}
If my understanding is correct, it's basically saying: if mPezCount is equal to 0, return boolean value TRUE. if mPezCount is not equal to 0, it will return false.
But when I try the following code on Example.java (and considering mPezCount is set to 0 in constructor)
public class Example {
public static void main(String[] args) {
System.out.println("We are making a new Pez Dispenser");
PezDispenser dispenser = new PezDispenser("Donatello");
String str = Boolean.toString(dispenser.isEmpty()); //trying to convert boolean to string
System.out.printf(str);
}
}
Isn't this supposed to print "TRUE"? I'm actually getting something else.. and this made me think about how boolean actually work.
Thanks!
2 Answers

Yonatan Schultz
12,045 PointsYour understanding is fine as I read it. I think the issue is with your String str = Boolean.toString line.
If you want to create a String with the value of your boolean dispenser.isEmpty() I think you want to use:
String.valueOf(dispenser.isEmpty());
As per the String class documentation here:
http://docs.oracle.com/javase/7/docs/api/java/lang/String.html

Apoorva Shukla
9,138 PointsHi Ivan,
I tried Boolean.toString() function in my code and it is working fine. Have you declared mPezCount = 0 in your constructor method. My code is:
public class Example {
public static void main(String[] args) {
// Your amazing code goes here...
System.out.println("We are a making a new Pez Dispenser.");
PezDispenser dispenser = new PezDispenser();
String str = Boolean.toString(dispenser.isEmpty());
System.out.printf("Is dispenser empty: %s\n",
str);
}
}
This is my constructor method: public PezDispenser(){ mPezCount = 0; }
This is my isEmpty method: public boolean isEmpty(){ return mPezCount == 0; }
May be this code will provide some help to you
Thanks!