Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial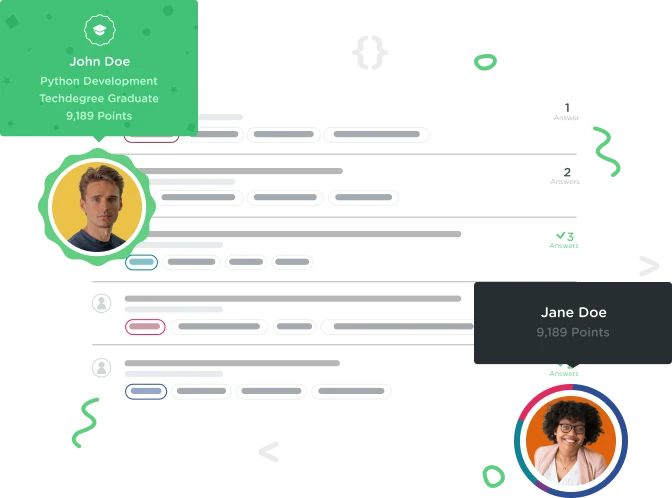

Nitin Chauhan
Courses Plus Student 277 PointsHow does hit_points become an optional argument when it has not been passed as a key to kwargs?
In the video, Kenny passed hit_points as an argument to make the hit_points of the new instance something other than the default value. But we have not specified hit_points as a key of kwargs. Then how does Python know that hit_points is the new key. The reason I am confused has something to do with the following code as well:
class Monster:
def __init__(self, **kwargs):
self.hit_points = kwargs.get("hit_points", 5)
self.color = kwargs.get('color', "yellow")
self.weapon = kwargs.get("arsenal", "kungfu")
self.sound = kwargs.get("sound", "roar")
def battlecry(self):
return self.sound.upper()
In this code, if I create a new instance, and pass weapon as an argument, say
fire_devil = Monster( weapon = "chupwock")
This doesnt't work. It didn't throw any error, and that was surprising too.
However, when I do this,
fire_devil = Monster(arsenal = "chupwock") It works as expected The instance fire_devil is created with weapon as "chupwock". I understand that this happens because "arsenal" is a key of the dictionary kwargs.
But in the code shown in the current video, we have not specified the keys of the dictionary kwargs. Then how does Python know about them? Does it take the attribute name as the key?
1 Answer

Nitin Chauhan
Courses Plus Student 277 PointsBut how does the code which key to associate to which attribute. I mean what are these default values.
For e.g. when I am saying something like
fire_devil = Monster(experience = 5)
Do you mean that this will associate a value of 5 to self.experience because the attribute is named so?
I hope I am making myself clear. In case I am not, do you mean something like this
instance = Class(some_attribute = num)
and this will associate the value num to some attribute named self.some_attribute?
I am sorry I am not able to understand this.
Cheo R
37,150 PointsCheo R
37,150 PointsYou can pass a default value to get() for keys that are not in the dictionary. That's why
fire_devil = Monster( weapon = "chupwock")
doesn't throw an error. The code
self.weapon = kwargs.get("arsenal", "kungfu")
says, get me "arsenal", if it't not there, then get me the default value "kungfu".