Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial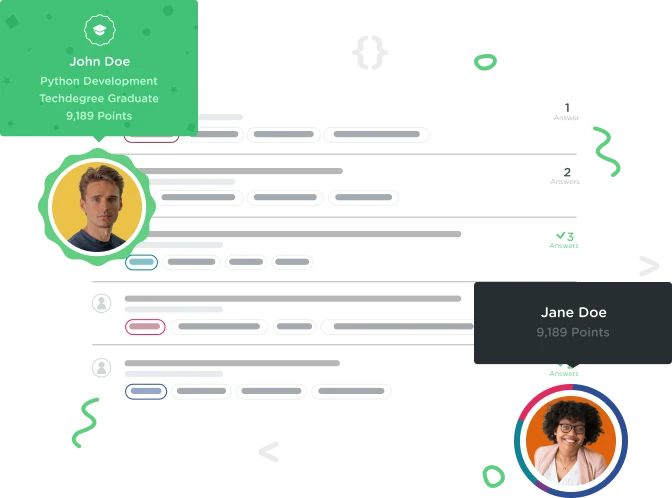

Ivan Yudhi
2,031 PointsHow does IllegalArgumentException work?
I don't quite understand how the following work:
From PezDispenser.java:
if (newAmount > MAX_PEZ) {
throw new IllegalArgumentException("Too many PEZ!!!");
}
From Example.Java
catch (IllegalArgumentException iae) {
System.out.println("Whoa there!");
System.out.printf("The error was: %s\n", iae.getMessage());
}
What is actually happening with throw new IllegalArgumentException("Too many PEZ!!!");
?
How does that relate to the catch block on Example.java being able to display the message when iae.getMessage() gets called?
Thanks.
2 Answers

Axel McCode
13,869 PointsBasically what "throw new illegal argument exception" does, is it throws an exception when a method is unable to resolve that exception on its own. This exception must be caught, so in my example below the run() method throws an Illegal argument exception. So think of it like this: the run() method threw the exception to the main method and the main method must catch it or else the program will crash. In the try-catch block an Exception object is set as the argument.
In other words, the catch block receives the IllegalArgumentException from the run() method and then "exception.getMessage()" retrieves the message/String that details what the exception was and it is printed to the console.
Example:
public class MyClass {
public static void main(String[] args){
MyClass MC = new MyClass();
try {
MC.run();
} catch (IllegalArgumentException exception) {
System.out.println(exception.getMessage());
}
}
public void run() throws IllegalArgumentException{
/* random code that results in an illegal argument exception being thrown*/
}
}
Hope this helps :)
-Axel

Ivan Yudhi
2,031 PointsHow does the catch block knows which message to get pulled if you have more than one 'throw' scenarios (I hope that's clear enough.. not sure how to explain)?
Back to my example, there's only 1 throw statement in the PezDispenser code. what if there's more than 1? How do you know which one to catch when doing the try catch block?

Axel McCode
13,869 PointsWhen you have more than one exception thrown you can just simply add more catch blocks to deal with them.
Take a look at the code below:
If the run() method throws an IOException, then the IOException catch block would run, same with the IllegalArgumentException.
Now, as you can see there is a third catch block. This catches any other Exception that is thrown by the run() method. So if a RuntimeException is thrown both the IO and IllegalArgument Exceptions can't catch it because they are both different exception types. But that last catch block with a Exception object as its argument has the ability to catch any type of exception thrown by the run() method.
Usually your IDE will give you an error notification when you have code that could result in an exception type having to be dealt with. This is how you know what exceptions you should catch, and if this is not the case, run your program and check the logs/console if you get an error/crash. The information displayed there can tell you whether it was caused by an Exception.
Here is a helpful site that helped me better understand exception handling. http://www.tutorialspoint.com/java/java_exceptions.htm
public class MyClass {
public static void main(String[] args){
MyClass MC = new MyClass();
try {
MC.run();
} catch (IllegalArgumentException exception) {
System.out.println(exception.getMessage());
} catch (IOException exception) {
System.out.println(exception.getMessage());
} catch (Exception exception) {
System.out.println(exception.getMessage());
}
}
public void run() throws IllegalArgumentException, IOException, RuntimeException{
/* random code that could throw various types of Exceptions*/
}
} // end class