Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial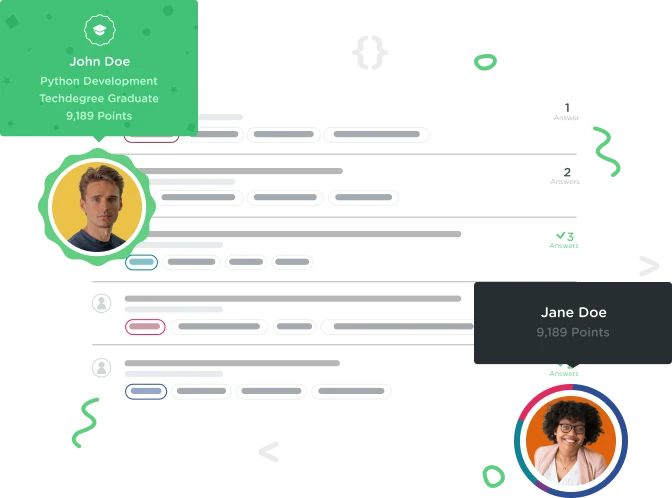

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsHow does instance know to call its prototype?
In the video, while the prototype.roll is outside of constructor and when a new instance is called e.g
Var foo = new Dice(10);
How come the prototype roll worked, as Roll was outside of Dice,

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsLoris Guerra sorry i didn't understand.
3 Answers

Michael Braga
Full Stack JavaScript Techdegree Graduate 24,174 PointsWhat happens is that when you call on foo.roll() the JavaScript interpreter will try to call roll method on the object itself which in this case is the Dice object assigned to var foo:
function Dice(sides) {
this.sides = sides;
}
//did not find roll method here
Since the interpreter did not find the roll method, the next thing it does is go to its prototype object and try to located it there:
Dice.prototype.roll = function() {
return Math.floor(Math.random() * this.sides) + 1;
};
//found roll method here!
Since the roll method is found within the Dice.prototype it will now call the method in the context of that instance. So with Var foo = new Dice(10);
this.sides will be 10 within the roll method because thats the instance that we called it on.
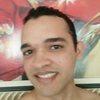
Rafael silva
23,877 Points1 - I will make for you my solution first step in the work space type something like this inside dice.js:
function Dice(sides){ this.sides = sides; this.roll = function (){ var randomNumber = Math.floor(Math.random() * dice.sides)+1; return randomNumber; } }
var dice = new Dice(6);
2 - the second step you need to type something like this inside ui.js
function printNumber(message){ var placeholder = document.getElementById('placeholder'); placeholder.innerHTML = message; }
var button = document.getElementById('button');
button.onclick = function (){
var result = dice.roll();
printNumber(result);
};
if do the same as share with you , will work and I hope help you.

nico dev
20,364 PointsBecause a) When you create an instance, you're telling it to inherit properties from the Constructor (i.e.: when you use the new keyword, you're saying "I want you instance X to inherit properties from Z Constructor", like in:
//supposing you already created a Constructor function Bar
var foo = new Bar(10);
Well, there you are telling foo to inherit the properties of Bar Constructor.
b) You can always add properties and values to an instance or a constructor. Like:
foo.myProperty = "Hi there!"
c) Having said that, you can also add a property/value to the prototype property, which will basically add it to every instance you create from now on made of that Constructor.
So if you do:
Bar.prototype.myInherProp = "Good bye!"
// If you call now the foo.myInherProp value, it will return you "Good bye!"
d) I guess you are asking about scope, but the scope of the prototype is from the constructor function all the way down through the prototype chain.
Hope that helped!
Loris Guerra
17,536 PointsLoris Guerra
17,536 PointsWhen you define the "roll" function, you add it to the PROTOTYPE of Dice. If you try to inspect "foo" on your browser's console, you'll see that the function is added inside the "__ proto __" key.
So, when "foo" tries to access its "roll" method and won't find it, "foo" will try to search it down to the prototype chain, inside "__ proto __" , THAT IS WHERE YOU ADDED THE "ROLL" METHOD --> Dice.prototype.roll = function(){ .... }
I hope this helps.