Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial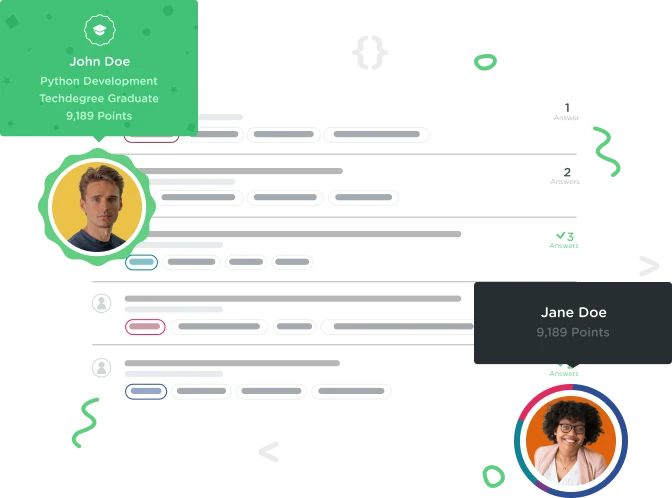
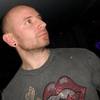
Philip Bradbury
33,368 PointsHow does it know what to compare?
So im struggling with the compareTo in this code..
@Override
public int compareTo(Object obj) {
Treet other = (Treet) obj;
if (equals(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
when we use compareTo in this class how does the Object parameter come into play? how does it know that parameter is another specific Treet (i dont mean as in the type treet) Im seeing it as this, how does it know that object is a second treet?
@Override
//compare this treet to treet2(wheres does it know to look the treet2)
public int compareTo(Treet treet2) {
//if this tweet equal treet2
if (equals(treet2)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(treet2.mCreationDate);
if (dateCmp == 0) {
return mDescription.compareTo(treet2.mDescription);
}
return dateCmp;
}
hope I've explained what I'm getting at Say i have two footballs in front of me and i want to compare football1 to football2, so i say football1.compareTo(football2), simple. But this is saying i wanna compare football1 to another object I've not told you about so, football1.compareTo(object). I know i want that object to be football2 but how does java know that if I'm not pointing it to football2?
3 Answers
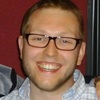
Victor Learned
6,976 PointsThe compiler checks the type at compile time to ensure that it is getting the correct type. So when you are passing the Object obj you are immediately attempting to cast it to a Treet on your 1st line of the compareTo method (Treet other = (Treet) obj;). This is where it's checking. If it can't cast it should throw an exception and then you'll know it's not a Treet obj.
The way you are seeing it in your second segment of code is not how it is really happening. Though honestly that would be the better way to do it in my honest opinion. It makes it easier for readability, code reviews and for future maintainability.
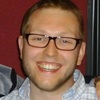
Victor Learned
6,976 PointsSo are you asking about how the Arrays.sort(treets) is working with the compareTo method? Or are you still confused by Object obj being passed? If you are still confused my question to you is: do you understand what the Object class is in Java?
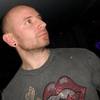
Philip Bradbury
33,368 PointsI understand how the obj is working in the compareTo method, just not how java makes obj equal to secondTreet's data(or any other treet object created). I understand the Object class as the top of the hierarchy in java a superclass as such.
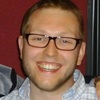
Victor Learned
6,976 PointsYou just answered your own question: "Object class as the top of the hierarchy in java a superclass as such". Thus EVERY object in Java is a child of Object. That's how obj equals the secondTreet. SecondTreet is an Object since it's a child of the Object class. Thus in Java if a method accepts a superclass (or parent) then it will also accept its subclass (or child which is a Treet in this case). Since the compareTo method accepts an Object, you are free to pass in whatever you want, provided that it is not one of the primitive types. There is no way to verify, at compile time, how the class is used. That's why they do a cast.
Read this https://docs.oracle.com/javase/tutorial/java/generics/types.html Read this on Generics and inheritance: https://docs.oracle.com/javase/tutorial/java/generics/inheritance.html
Joshua DeJong
3,138 PointsI am not sure if you ever resolved this Philip, or if it has completely passed out of your mind by now but it seems that the issue has to do with the fact that the compareTo() method is defining the class's behaviour and not implementing that behaviour. You would implement that behaviour somewhere else by instantiating two Treets and then use the compareTo method directly, or indirectly by sorting in an array.
For example:
public static void main(String[] args) {
Treet treet1 = new Treet(//data here);
Treet treet2 = new Treet(//data here);
// Now you are set up to use the compareTo() method that was defined
// in the Treet class. The two treets are instances of the class with data
// that you supplied that can now be compared.
System.out.println(treet1.compareTo(treet2));
}
Hopefully that answers the original question, which I know is a little bit old now.
Cheers.
Philip Bradbury
33,368 PointsPhilip Bradbury
33,368 PointsSorry i don't mean the type that the obj is but how does it know where to get the data for the second treet to compare. So how does the system know that we we say object obj it knows to compare treet one with treet 2. We cast the type so it know obj will be a treet but how does it know which treet we what to compare it to. Say i have two footballs in front of me and i want to compare football1 to football2, so i say football1.compareTo(football2), simple. But this is saying i wanna compare football1 to another object I've not told you about so, football1.compareTo(object). I know i want that object to be football2 but how does java know that if I'm not pointing it to football2?
Victor Learned
6,976 PointsVictor Learned
6,976 PointsSo you are passing the object to the method. Thus the compiler now has access to that object and then can act on its data.
It's a little more complicated but you should understand how Java passes data to methods. A good post about it can be found here: http://stackoverflow.com/questions/40480/is-java-pass-by-reference-or-pass-by-value
Philip Bradbury
33,368 PointsPhilip Bradbury
33,368 PointsThank you for helping me with this. Ive read the stacker flow and i think i get it but i still get get this correct in my head.
Its this first part I'm struggling with, i get how the method is working just not how its getting the correct information to work with. The passing the method the information, how does the obj relate to say secondTreet in the example file. I see it as just saying is an Object type called obj but that doesn't relate to any information anywhere else so how does it know its talking about the trees objects set up in example??