Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial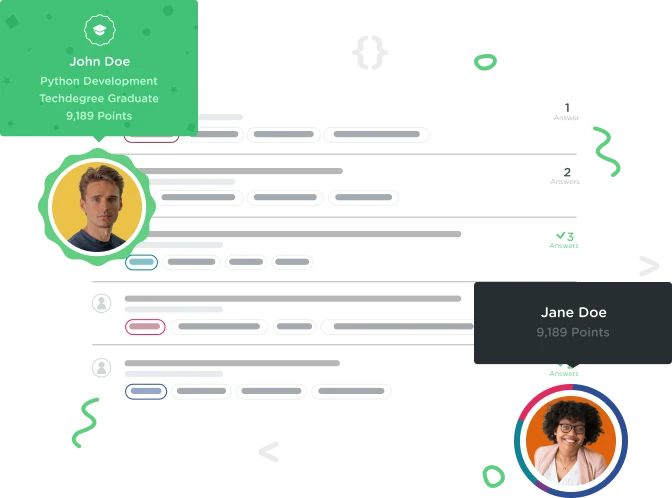

Idan Melamed
16,285 PointsHow does jQuery execute this line?
So, we got this code:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//Hide hints
$("form span").hide();
function passwordEvent(){
//Find out if password is valid
if($password.val().length > 8) {
//Hide hint if valid
$password.next().hide();
} else {
//else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if($password.val() === $confirmPassword.val()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//else show hint
$confirmPassword.next().show();
}
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).focus(confirmPasswordEvent).keyup(confirmPasswordEvent);
How does jQuery execute this line?
$password.focus(passwordEvent).keyup(passwordEvent).focus(confirmPasswordEvent).keyup(confirmPasswordEvent);
5 Answers
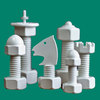
Steven Parker
229,786 PointsI'm not sure what you mean, but I will guess that you are confused by the methods being attached to each other.
Each of these method calls pass back the element they acted on as their return code, so that one line is essentially a shorthand for these four separate lines:
$password.focus(passwordEvent);
$password.keyup(passwordEvent);
$password.focus(confirmPasswordEvent);
$password.keyup(confirmPasswordEvent);
This programming technique is known as method chaining or method cascading.
An event listener is like an alarm clock - once you set it, you don't have to keep checking - it will let you know when the time comes. That's why when you establish a listener you give it a "callback function". The browser or interpreter will run the callback function when the event occurs. In the meantime, your program can do something else, or there may be no active code running at all (this is typical).

Idan Melamed
16,285 PointsThanks Steven, Thanks for the explanation.
Yeah, things are now clearer, and it answers half of my questions.
Here's something I still don't get, and I'm not sure I articulate my question well enough...
A language program usually goes through every line from top to bottom and decides whether to run a line or not (I'm over simplifying things here). In this example, it seems to me like jQuery has to run these event listeners repeatedly to check if the users changes anything.
How does this happen? Maybe what I'm asking is how does the interpreter or browsers execute event listeners?
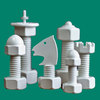
Steven Parker
229,786 PointsRunning something repeatedly to check is called "polling". That's the opposite of an event-driven system. I added a paragrph about that to my original answer above.

Idan Melamed
16,285 PointsThank Steven, Really cleared it up for me!
The more I know about browsers, the moreI realize how much I don't know. :-)

Ian Friedel
11,960 PointsSteven Parker when you answering a question how do you create the black box to show your code?

Idan Melamed
16,285 PointsYou put a ``` before it and another one after it
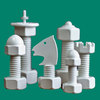
Steven Parker
229,786 Points I got your request.
Thats blockquoting, as described in the Markdown Cheatsheet pop-up link down below
Skip a blank line, then a line with three accents (```), then your code, then another line with the accents (```). Put the name of the language after the first set of accents to get syntax coloring. Like this:
```javascript
(or just "js")
(your code goes here)
```

Ian Friedel
11,960 PointsSteven Parker thank you for the response! I didn't see the markdown cheatsheet before this will be extremely helpful in the future!