Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial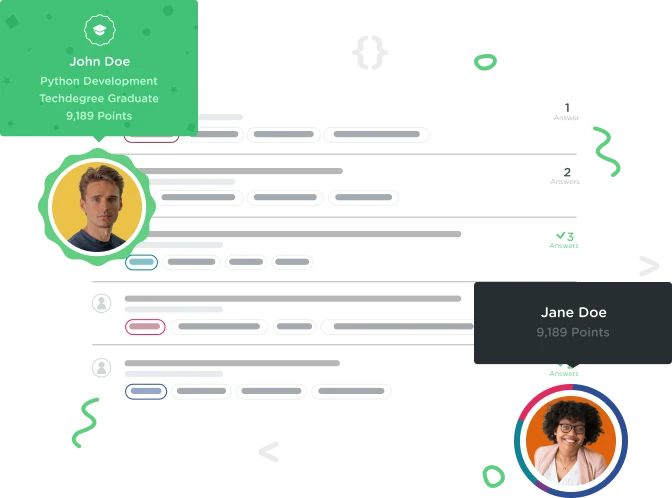
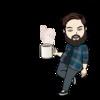
Philip Schultz
11,437 PointsHow does memory work in Python?
Hello everyone, I'm finding it difficult to understand what exactly is happening when we make variables and copy variables in python (and list, dictionaries, etc), also testing them in conditional statements. After doing some research on my own I think I narrowed my question down to be "What is the difference between passing by reference and passing by value". Can someone show me an example of what exactly the difference is between the two and what is going on in the background with memory? Also, How do you know when to use the double equal or the 'is' and 'is not' operators in conditional statements? Are there major differences between high-level languages in regards to this, or are they all the same.
1 Answer
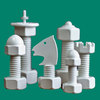
Steven Parker
241,783 PointsThe concepts of "passing by reference" and "passing by value" are used in other languages but don't really apply to Python. Arguments in Python are "passed by assignment" which can appear to be either of the other types depending on the circumstances.
The important concept in Python is that everything is an object, and objects have both identity and value. Identity is essentially the storage location in memory. The "is" operator compares identity and the "==" operator compares value.
Here's an example function that uses both tests to compare two things:
def compare(a,b):
if a is b:
print("a and b are the same object")
elif a == b:
print("a and b have the same value")
else:
print("a and b are completely different")
So, using that function you can show the difference between equality and identity:
>>> a = 12345
>>> b = a
>>> compare(a,b)
a and b are the same object
identity
>>> b = 12345
>>> compare(a,b)
a and b have the same value
equality
And this is not typical of other languages.
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsSteven, I thought I understood, but now I'm confused again. I've been playing around in the python shell and I found some very weird things. I found that if I have two strings that are equal then they are considered the exact same object, but only if they don't have any spaces in them. For example.
What is going here? Also, for numbers.
Why are equivalent strings and numbers the same object, but only up to a certain point?
Steven Parker
241,783 PointsSteven Parker
241,783 PointsNow you're seeing the effects of internal optimizations. Small integer values (256 or less) and some strings will be combined and share memory. It can do this safely because numbers and strings are both immutable.
I don't know enough about how the optimizer combines strings to explain why it doesn't do it when the string has spaces. Just be aware that Python does do this sometimes in an effort to be efficient.
"Pay no attention to that man behind the curtain!"
Philip Schultz
11,437 PointsPhilip Schultz
11,437 PointsCool, Thanks again