Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial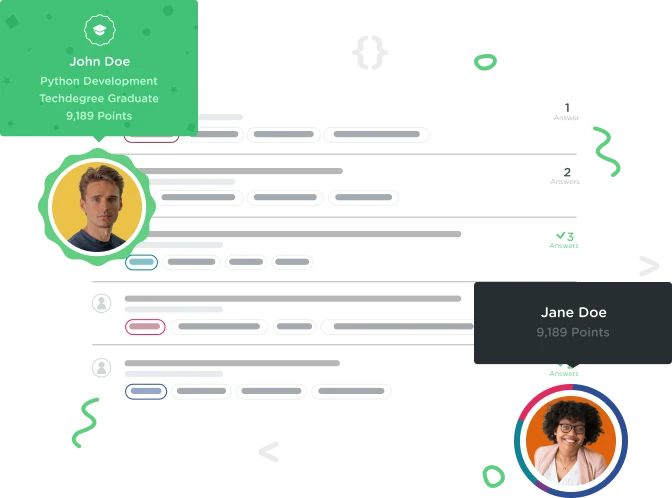
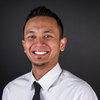
Miguel Agawin
3,211 PointsHow does one variable target another array?
In the tutorial, the value of a string in one array variable was accessed by a second variable (new line; different name) by simply inputting a number (var friendsName = 1) that somehow pointed to the index within the array of the first variable. How does it know that that number is first of all an index and how does it know to target specifically the array of the previous variable?
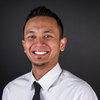
Miguel Agawin
3,211 Pointsvar friends = ["Nick", "Michael", "Amit", "Allison Grayce"];
var friendNumber = 1;
console.log(friends[friendNumber]);
6 Answers
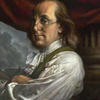
Jeff Busch
19,287 PointsHi Miguel.
All that's being done here is friendNumber is being assigned the value of 1. The following two lines of code would be equivalent.
console.log(friends[friendNumber]); console.log(friends[1]);
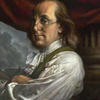
Jeff Busch
19,287 PointsMiguel,
friendNumber doesn't know anything except that it is the number 1. Copy the code below and paste it into a text editor and save it as an html file, then open it in a browser. Now play with the code simply by changing the value assigned to friendNumber. Save the file and refresh the browser, Hopefully this will help you understand what's happening.
Jeff
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html" charset="utf-8" />
<title>Test Javascript Variables</title>
</head>
<body>
<script type="text/javascript">
var friends = ["Nick", "Michael", "Amit", "Allison Grayce"];
var friendNumber = 1;
console.log(friends[friendNumber]);
console.log(friends[1]);
document.write(friends[friendNumber]);
document.write("<br>");
document.write(friends[1]);
</script>
</body>
</html>
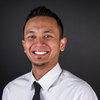
Miguel Agawin
3,211 PointsMuch appreciated. Many facepalms to come.

Stone Preston
42,016 Pointsok so you have an array called friends. You can access elements of the array using the array name followed by a square bracket followed by the index of the array you want followed by a closing square bracket. Remember array indexes start at 0.
so friends[0]
is "Nick" friends[1]
is "Michael" and so on.
you then set another variable friendNumber to 1.
then log to the console friends[friendNumber]
But what is the value of friendNumber? its 1. so that line can be translated to console.log(friends[1]);
which will output "Michael"
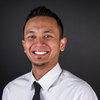
Miguel Agawin
3,211 PointsI understand what it's doing, I just don't know how the second var knows to communicate with the previous one but I guess that's the way JS works – whatever array (of, say, multiple array variables) is placed immediately before a variable that points to an index will be the array that that following variable targeting an index will be pointing/targeting to. Two subsequent tutorials confirm this and it was indeed touched upon in an earlier tutorial dealing with object keys/properties – how their values can be altered by new ones written outside (in a lower line).

Stone Preston
42,016 Pointsare you talking about friends[friendNumber]? what do you mean by " how the second var knows to communicate with the previous one"
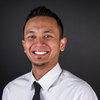
Miguel Agawin
3,211 PointsYes. I don't know how friendNumber knows to look in an array variable called friends. What if there were three arrays – family, friends, colleagues. How does a new var (like friendNumber) know to target index 1 specifically from the friends array? My assumption is friends would have to be placed immediately before friendNumber or at least be the last array written before friendNumber.

Stone Preston
42,016 PointsMiguel Agawin, friendNumber doesnt know to look in an array variable called friends. Its just a number. your assumptions about what gets placed immediately before this and that are not correct. How array indexing works is like this. You have an array called someArray. lets say its declared like this.
var someArray = ["hello", "im", "an", "array"];
to acces the members of that array you can use array indexing. If wanted to access the member "hello" i would use someArray[0]
if I wanted to access "array" i would use someArray[3]
. Now lets say I want to use a variable as the index instead of a hardcoded number. I would declare a new variable named index like so: var index = 2;
now we have a variable whose value is 2. thats it, just a number. now I want to access a member of someArray using that variable called index. i would use someArray[index]
which is really the same thing is saying someArray[2];

Stone Preston
42,016 Pointshere is a code example
var friends = ["Nick", "Michael", "Amit", "Allison Grayce"];
var index = 2;
var otherArray = ["Amit", "Allison Grayce", "Nick"];
console.log(friends[index]);
console.log(otherArray[index]);
it does not matter what array comes before this and that. index is just a number (2 in this case) so the console would show "Amit" from the friends array and "Nick" from otherArray
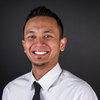
Miguel Agawin
3,211 PointsYes yes, I fully understand now. I overlooked that friendNumber is simply 1. Thank you both!

Stone Preston
42,016 Pointsalright cool. its a concept that comes up again and again so its important to understand how it works and how to use it.
Stone Preston
42,016 PointsStone Preston
42,016 Pointscan you post some code. Its hard to see what you are describing without some context