Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial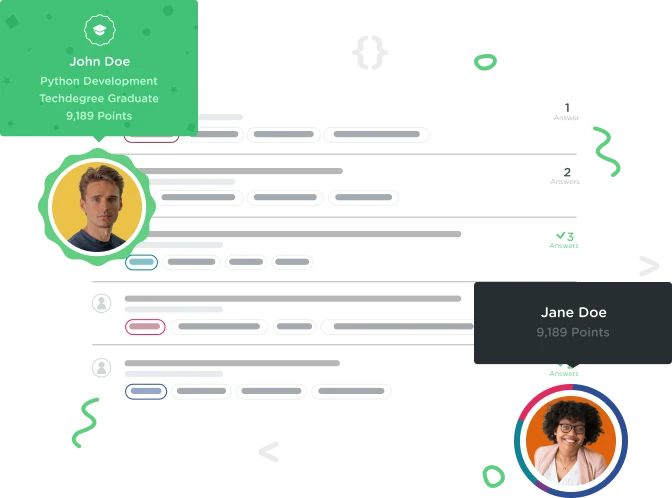

nicholasdevereaux
16,353 PointsHow does python know if the number is even or odd by this IF statement: if even_odd(number):
import random
start = 5
def even_odd(num): return not num % 2
while start: number = random.randint(1, 99) if even_odd(number): print("{} is even".format(number)) else: print("{} is odd".format(number)) start -= 1
2 Answers
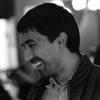
Martin Cornejo Saavedra
18,132 Pointseven_odd(number) is a function that uses the modulo operator '%' to calculate the residual of the number. It is well know that even numbers have residual equal to 0, and odd numbers don't. That's why when the remainder is 0, the 'not' operator negates the 0, thus returning a 'True'(true, it is even) statement (oposite of 0 is 1 or True in python). Else, it returns a 'False' statement.
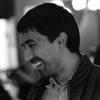
Martin Cornejo Saavedra
18,132 Pointsnicholas, as lambda says, it isn't a built-in function, it is a custom one, the code that defines the function is the one you posted:
def even_odd(num): return not num % 2
nicholasdevereaux
16,353 Pointsnicholasdevereaux
16,353 PointsOkay thank you! I didn't know even_odd is a built in function. I thought it was just a random name given to the function.
lambda
12,556 Pointslambda
12,556 Pointseven_odd isn't a built-in function. It's a function that you write. The modulo operator % is how you can tell if a number is odd or even and that is built into python.