Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial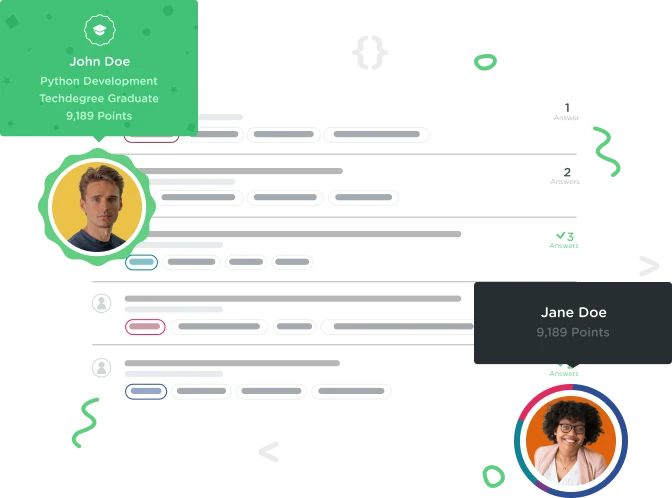
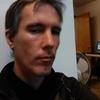
Mark Miller
45,831 PointsHow does python know where the end of a for-loop code block is? There's no indication of when execution should restart.
I can't use "continue" or "break" because the code block should execute for each item, and then break when no further items are available. The code block in a for loop (in python) has no curly braces nor an "end" keyword indicating the point where the block terminates. All other languages have the code block wrapped in some way to move execution back to the top for each item.
def disemvowel(word):
vowels = ['a', 'e', 'i', 'o', 'u', 'A', 'E', 'I', 'O', 'U']
container = list(word)
for letter in vowels:
try:
container.remove(letter)
except ValueError:
pass
new_word = ''.join(container)
return new_word
1 Answer
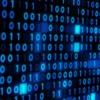
Alexander Davison
65,469 PointsYou have to use indentation to represent something is for something else:
for x in range(10):
# all code indented are in the for loop
print("This is *in* the for loop!")
print("So as this!")
print("This is OUTSIDE of the for loop because it isn't indented.")
This is just a simple example, but it can get more complicated:
for x in range(10):
# This is in the loop
print("We are currently on the number {}!".format(x))
if x == 5:
# This is in the if statenent which is in the loop
print("Cool! 5!")
if x % 2 == 0:
# This is in a different if statement but still in the loop
print("Alright! {} is an even number!".format(x))
# This is in the for loop but not in a if statement
print("Next number coming up... {}!".format(x+1))
# This code isn't in any kind of block
print("Finished!")
This is why Python is so picky about indentation. If you forgot to indent like this:
for x in range(5):
if x == 5:
break
Python will cause an Syntax Error because it thought you put nothing in the if
statement and there has to be something. Python also will think that the break
command is part of the loop, but we really don't mean that. Keep in mind that you have to be very careful indenting when you are a beginner! You get used to it when you get more advanced :)
I hope this helps. ~Alex
Mark Miller
45,831 PointsMark Miller
45,831 PointsThanks. I did not know indentation has meaning.
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsNo problem :)