Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial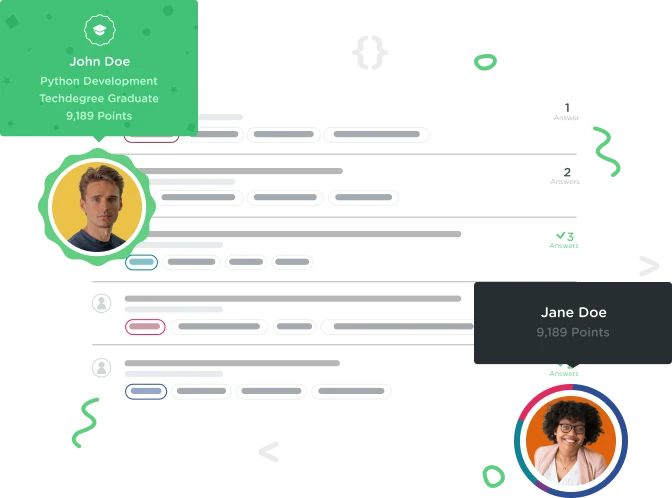

Charp jay
177 Pointshow does the application determine the next page based on which button is clicked? please show me the code related ?
can anyone please show me the code that explains the logic to the computer as to which page to get when button 1 is clicked and which to get when button 2 is clicked?
2 Answers
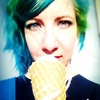
Sara Chicazul
7,475 PointsThe code that controls which page the buttons load is in the 'onClickListener' event for each button:
// Button 1
mChoice1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// When button is clicked, get the NextPage value from the Choice1 object
int nextPage = mCurrentPage.getChoice1().getNextPage();
loadPage(nextPage);
}
});
Remember, each Page stores two Choice objects. A Choice object contains the text on the button for that choice, and an integer representing the next page to load. When the button is clicked, we get the NextPage value and pass it into the loadPage() function.
private void loadPage(int pageIndex) {
// Get page whose index matches the parameter pageIndex
mCurrentPage = mStory.getPage(pageIndex);
// Update text and images using values from current page
}
This works because we have stored the Pages as an array in mStory, so each page can be accessed by a unique integer.
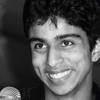
Dhruv Gupta
4,320 PointsI did this course a while ago, here:
Go to your Story.Java
I made mine so it reflected a societal issue :P
public Story() {
mStory = new Page[9];
mStory[0] = new Page(
R.drawable.page0,
"The American Rich were an extremely wealthy class. The wealthiest out of all the rich people in the world. The top 1 percent of Americans held more wealth than the bottom 90 percent of Americans combined. Yet despite the enormous amount of wealth, they were greedy for more!",
new Choice("Live your life working for these individuals", 1),
new Choice("Live your life protesting against these individuals", 4)
);
mStory[1] = new Page(
R.drawable.page1,
"The one percent had made the fateful mistake that led to the downfall of Rome. They made material goods the source of personal fulfillment. In their mad quest for resources they sent spaceships to Mars to mine the planet for precious resources.",
new Choice("Be a passive observer", 2),
new Choice("Join the resistance movement", 5)
);
mStory[2] = new Page(
R.drawable.page2,
"They had not expected that an advanced subterranian society lived beneath the surface of Mars. The Martians having felt the greed of the 1 percent resolved to invade the planet and turn the bodies of the one percenters into fuel",
new Choice("Be a passive observer", 3),
new Choice("Join the Alien Army", 4)
);
mStory[3] = new Page(
R.drawable.page3,
"Before their invasion they have abducted you to tell them the 1 percent's weaknesses. You them that human beings have a general angst due to existence. They cannot wrap their heads around it.",
new Choice("Assist in whatever manner possible", 4),
new Choice("Go home", 6)
);
mStory[4] = new Page(
R.drawable.page4,
"You are hereby chosen by Aliens on Mars to use their spaceships to do destroy the one percent. You go to the ship and records 'Everyone will eventually die. The difference is as you lay dying you will look back on a disgusting apathetic life'",
new Choice("Go to Earth", 6),
new Choice("Wait for the Alien Reaction", 5)
);
mStory[5] = new Page(
R.drawable.page5,
"The aliens are startled that such a statement could possibly be novel to the one percent, but after conducting further observations concluded that you are correct. The cute ragmuffins bid you farewell with their spaceship that could transmit the statement directly to every one percenter",
new Choice("Go to Earth", 6),
new Choice("Destroy the one percent", 7));
mStory[6] = new Page(
R.drawable.page6,
"You arrive on Earth. You have come to a deserted woodland in a spaceship capable of ending the 1 percent. Questions about life ring. Who are you to make a judgment call? Would you have been different had you been part of the 1 percent. Are the 1 percent in anyway necessary for society to functino?",
new Choice("Kill them ALL", 7),
new Choice("Take a ten billion dollar bribe", 8));
mStory[7]= new Page(
R.drawable.page6,
"You decided that the entire top 1 percent of the population deserves extermination. Every Government on Earth is wiped out. After four generations of social upheavel everything is back to status quo. Except now the rich don't mess with Mars unless with they do it jointly with the Martian one percent! "
);
mStory[8]= new Page(
R.drawable.page6,
"So now you are one of them. You saw the opportunity and took advantage. It's not like luck had anything to do with it. So why, why would you help those outside the 1 percent. You worked to get to where you are. They're just lazy bums who don't sieze opportunities"
);
The choices are set in Choice.Java
public Choice getChoice1() { return mChoice1; }
public void setChoice1(Choice choice1) {
mChoice1 = choice1;
}
public Choice getChoice2() {
return mChoice2;
}
public void setChoice2(Choice choice2) {
mChoice2 = choice2;
}
Charp jay
177 PointsCharp jay
177 Pointsplease don't mind, your answer has been helpful but i still dint understand how the app decides that when the button 1 is clicked it has to get a particular next page?
Charp jay
177 PointsCharp jay
177 Pointsi mean, in the array we have specified them one below the other, but where is the logic that on clicking a particular button it must get a particular element from the array? hows it doing the logic?
Sara Chicazul
7,475 PointsSara Chicazul
7,475 PointsDo you see the first bit of code I posted? It starts out
mChoice1.setOnClickListener
.mChoice1
is the variable we created and set equal to Button 1. AnonClickListener
is a bit of code that "listens" for a certain event to happen, in this case: clicking on Button 1. When that happens, it runs the code inside the function.Is that any clearer?
Charp jay
177 PointsCharp jay
177 Pointsi understand everything but the only thing that i haven't understood is where have we specified that the app should reach out to a particular page if button 1 is clicked? i mean where is the integer representing the next page for a particular button click provided by us ? That would clear everything.
Sara Chicazul
7,475 PointsSara Chicazul
7,475 PointsThe integer representing the next page is selected with this line:
int nextPage = mCurrentPage.getChoice1().getNextPage();
The NextPage variable stores the index value of the next page.