Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial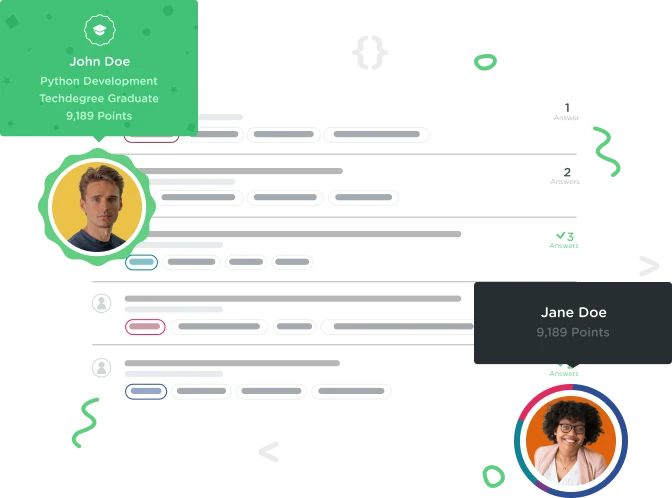

Peter Larson
6,412 PointsHow does the code know the text string is supposed to be passed into console.log?
How does this code know that "Greetings, everyone!" is what is supposed to be passed into the console.log output?
window.setTimeout((something)=>{
console.log(something);
}, 3000,'Greetings, everyone!');
2 Answers

andren
28,558 PointsWhen you call setTimout
with three arguments then the first will be treated as a function, the second a delay for that function to run, and the third a value you want to pass into that function as an argument.
Since 'Greetings, everyone' is the argument you pass into the function, and the function simply prints out its first parameter (which is assigned to the first argument) you end up with that value printed.
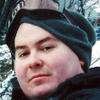
Jason Cook
11,403 PointsThe best way I can answer this question is to break it down. First, it's important to look at the syntax of the setTimeout() method.
Syntax: setTimeout(function, milliseconds, param1, param2, ...)
So, in your case, you define an arrow function expression, which becomes the first argument (in the syntax above), then you pass '3000' as the number of milliseconds to wait, before executing the function, then lastly you pass your parameter ('Greetings, everyone!') which gets assigned to something in your arrow function expression.
window.setTimeout((something)=>{
console.log(something);
}, 3000,'Greetings, everyone!');
So, after loading your code, you can use Ctrl+Shift+J on PC or Cmd+Opt+J (Mac), to load the javascript console in Chrome, and after a short 3000ms wait, you'll see your message in the console.
I hope this explains it well enough. Basically the important thing to realize is that you're passing an arrow function expression as your first argument to the setTimeout() method, then the time to wait in milliseconds as the second argument, then last you pass the parameters that get assigned to something in your arrow function.
Happy coding :)

Moe Ghashim
11,109 PointsThis explanation helps a lot. I have a question though. How did we assign the parameter "Greetings, everyone!" to the something

Johnny Harrison
4,331 PointsGot it. So basically, it works because window.setTimeout is designed to work that way. And it's doing... whatever it's doing in the background to feed the parameter into the function, behind the scenes.