Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial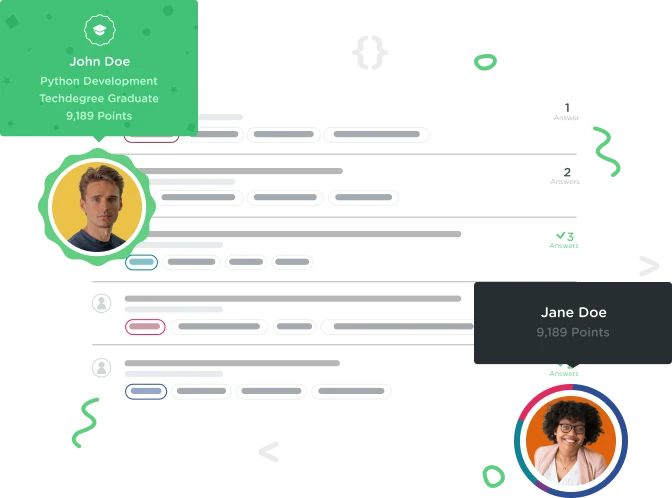
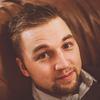
James Hammond
1,297 PointsHow does the code know what to actually get and set?
Ive just gone through the get and set video in C#.
I am wondering how does the program actually know what to get and set if thats all the code says to do?
like adding {get; set;} onto the end of something without directly saying what its getting and setting?
1 Answer

andren
28,558 PointsWhen you use the get
and set
keyword without specifying any code C# automatically creates a private anonymous backing field for the property, and sets up a default getter and setter method for the field.
Which means that this code:
class Person
{
private string name;
public string Name
{
get
{
return name;
}
set
{
name = value;
}
}
}
And this code:
class Person
{
public string Name { get; set; }
}
Is functionally equivalent with each other.
James Hammond
1,297 PointsJames Hammond
1,297 Pointsso you can access the get of Name to see what it is, but access set and change the default? like is it just a placeholder?
andren
28,558 Pointsandren
28,558 PointsI'm not sure I entirely understand your question. A property acts pretty much like a normal field variable except that the
get
andset
method is called automatically when the property is accessed or assigned. So you can access and assign it like this:With the default
get
andset
method that C# generates the property will act exactly like a field variable. If you define your ownget
andset
method then you can change what happens when it is accessed or something is assigned to it.James Hammond
1,297 PointsJames Hammond
1,297 PointsMakes sense now. Thanks!