Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial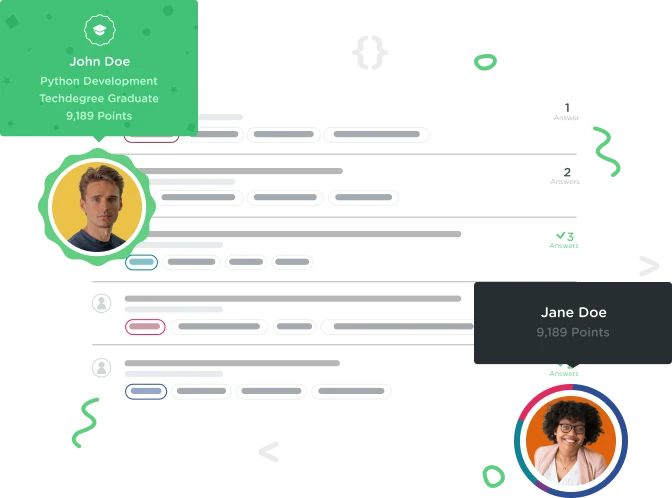
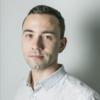
Kosta Kuts
15,894 PointsHow does the getJSON callback in the last eventlistener "know" what the data ( json ) it needs to execute?
Here's the sourcecode:
const astrosUrl = 'http://api.open-notify.org/astros.json'; const wikiUrl = 'https://en.wikipedia.org/api/rest_v1/page/summary/'; const peopleList = document.getElementById('people'); const btn = document.querySelector('button');
// Make an AJAX request function getJSON(url, callback) { const xhr = new XMLHttpRequest(); xhr.open('GET', url); xhr.onload = () => { if(xhr.status === 200) { let data = JSON.parse(xhr.responseText); // as soon as we got the data we execute callback return callback(data); } }; xhr.send(); }
function getProfiles(json){ json.people.map ( person => { getJSON(wikiUrl + person.name, generateHTML ); }); }
// Generate the markup for each profile
function generateHTML(data) {
const section = document.createElement('section');
peopleList.appendChild(section);
section.innerHTML =
<img src=${data.thumbnail.source}>
<h2>${data.title}</h2>
<p>${data.description}</p>
<p>${data.extract}</p>
;
}
//getJSON(astrosUrl);
btn.addEventListener('click', (event) => {
getJSON( astrosUrl, getProfiles ) event.target.remove(); });
1 Answer
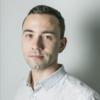
Kosta Kuts
15,894 PointsOk, I think I figured it out using the Chrome tool Sources -> Breakepoints
So basically when getJSON returns callback it sends param 'data', so it is how our 'json' is being send in the first place. Therefore there's no need to specify the argument and it is also not correct according to the syntax of fetching the callback function by its name.
what a relief.
Kosta Kuts
15,894 PointsKosta Kuts
15,894 PointsBefore we were sending json as a parameter to the callback, and I was following the flow:
//getJSON(astrosUrl); btn.addEventListener('click', (event) => { getJSON( astrosUrl, (json) =>{ json.people.map ( person => { getJSON(wikiUrl + person.name, generateHTML ); }); }) event.target.remove(); });
but now I'm confused because we are calling the callback without any arguments. How does it know what data to execute?!