Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial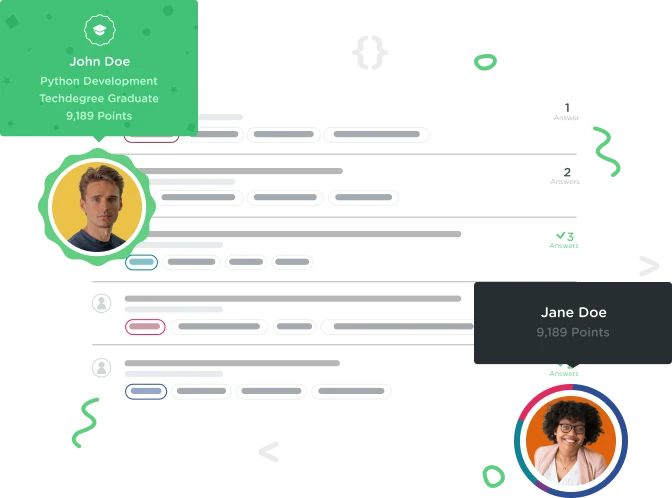
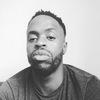
olu adesina
23,007 Pointshow does the => operator work in this forEach() method
how does the => operator work in this case never seen it used like this before
const fruits = ['apple', 'pear', 'cherry'];
fruits.forEach(fruit => console.log(fruit));
2 Answers
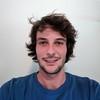
Jean-Luc Robitaille
13,864 PointsIt's a shorthand to write an arrow function.
let functionName = (parameters) => {
console.log("something");
}
In the shorthand syntax, if you have only 1 parameter, you can omit the parentheses. And if you only have 1 line of code, or only 1 action in your function body, you can write it without using brackets. So it becomes:
let functionName = parameters => console.log("something");
Check out the course teamtreehouse.com/library/getting-started-with-es2015-2 They talk about arrow function.

Juan Hurtado
2,243 PointsNormal way of doing a forEach
const fruits = ['apple', 'pear', 'cherry'];
fruits.forEach(function(fruit) {
console.log(fruit)
});
Using the => operator you are removing a few things from the way of writing the callback:
- function keyword
- parenthesis around the argument name
- curly braces surrounding the body of the callback e.g. console.log(fruit)
- replacing the callback console.log in the same line
Adding:
- => operator to denote what the callback returns
The purpose is to do the same but more concise.
Bear in mind both ways do the exact same thing