Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial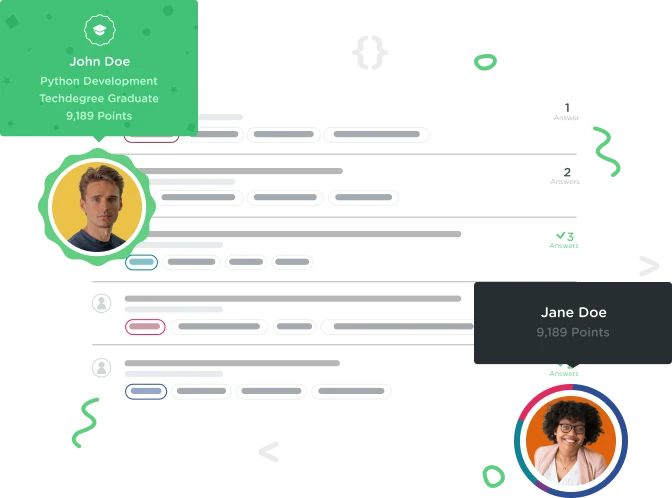
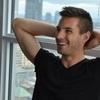
evanpavan
5,025 PointsHow does the return value in the Invader.Move method know to return false to the Level.Play method?
It seems counter intuitive as how the runtime would know which method to return the value to.
The Move method is a void method so it makes sense that nothing is returned to it in this case. But how does the runtime know to return the value of 'false' to the Play method when the return statement is nested in the Move method?
What if the Move method had a return type of bool? Would the return statement of 'false' within the Move method still return that value to the Play method in that case?
Is there something special here since this is the declaration of the Play method so any return statement nested within it will always return to the Play method and not the nested methods? What if one of the nested methods was also being declared rather than called and had a return type of bool?
Thanks for the help and following me through as I digress.
2 Answers

Luis Marsano
21,425 PointsBut how does the runtime know to return the value of 'false' to the Play method when the return statement is nested in the Move method?
Incorrect: the return statement is not nested in the Move method. It's just part of the next statement. Here's a better indentation:
public bool Play() {
// run until game ends (invaders neutralized or invader reaches end)
int remainingInvaders = _invaders.Length;
while (0 < remainingInvaders) {
// each tower has a chance to fire on invaders
foreach (var tower in Towers) {
tower.FireOnInvaders(_invaders);
}
// count and move invaders that survive
remainingInvaders = 0;
foreach (var invader in _invaders) {
if (invader.IsActive) {
invader.Move();
if (invader.HasScored) {
return false;
}
remainingInvaders++;
}
}
}
return true;
}
Focus on this
invader.Move();
if (invader.HasScored) {
return false;
}
The invader
is Move
d and then later checked (HasScored
); the outcome of that check decides whether to return false
.
- The "Move" statement changes the
invader
's state. - In the next statement, the "HasScored" expression checks that state.
If the
invader
"HasScored", thenreturn false
is evaluated. Otherwise, it's skipped.
Please mark the most helpful response as an answer to close this topic.

Emmanuel C
10,636 PointsIf I'm understanding your question correctly, the Move() doesnt directly affect how the Play() returns. That first return statement isnt nested in the Move(), It just looks that way from due to formatting. So as it goes through the loop, the invader moves, then you check if it that invader hasScored, which if i recall correctly, checks if the invader has reached the location of your base. But if it hasnt, then that return statement ,which is the return statement of the Play(), doesnt get called, so the remainInvaders increments and the loop starts over.
The Play() returns from which ever return statement gets called first. If the invader scores then the return false is called, however if the invader doesnt ever score through the loops and the towers can defeat the invaders, lowering the remaining invaders to 0 where that while loop finishes, then that return true is called. That keyword of return is directly responsible for how whatever method it is in returns and whichever gets called first within the lifetime of that method is how the method returns.
Even if Move() returned a bool, it wouldnt affect how Play() returns becaused its not being used as the value that gets returns by the return keyword. Its possible to have a method that returns something and not assign it to anything. so If move() return a bool the program wouldn't do anything with it since its not getting assigned to a variable. The only way Move() could affect how Play() is returned is if call on Play()'s return like this
return invader.Move();
This wouldnt make logical sense as Play() would return and end when that Move() is called.
Also you cant define a method inside of another method, atleast not in C#. I hope this makes sense to you and answers your questions. If it still doesnt make sense and you have more questions just post a comments Ill be happy to help you out.