Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial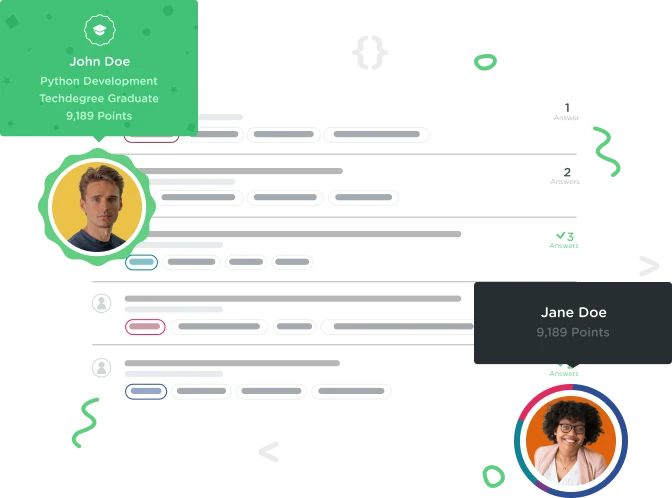

Kelvin Chen
5,152 PointsHow does the server know the request HTTP verb
The video explains that making request from Postman with PUT or POST requests. But if I am making the PUT or POST request from the browser with the same routing, how does the server know what kind of request I am making? And how do I send a JSON object with my requests in the browser?
3 Answers

Zimri Leijen
11,835 PointsExpress abstracts those things away, but whenever you make a request to the server, a lot of data is sent, along with that data is a header, which contains information like the type of request ('PUT', 'GET', etc..).
By default, a request is a 'GET' request, but when you make an interactive element on a website (usually a form or a button) you can specify the type of request being sent.
A form is often a 'POST' request for example (but not always).
I believe this course gives a bit more insight into it.
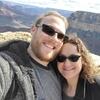
James Crosslin
Full Stack JavaScript Techdegree Graduate 16,882 PointsOn the client side, we include the type of request that's happening in our code. Let's show a simple XMLHttp get request:
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function() {
if (this.readyState == 4 && this.status == 200) {
// Typical action to be performed when the document is ready:
document.getElementById("demo").innerHTML = xhr.responseText;
}
};
xhr.open("GET", "filename", true);
xhr.send();
You can see here that in our request, we explicitly state that it's a "GET" request. We could change that value to post and include our json info. Or if you're more used to fetch:
fetch('https://example.com/profile', {
method: 'POST', // or 'PUT'
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify(data),
})
.then(response => response.json())
.then(data => {
console.log('Success:', data);
})
.catch((error) => {
console.error('Error:', error);
});
This way, we let the server know what kind of request we are making.
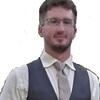
Sean Paulson
6,668 Pointshttps://en.wikipedia.org/wiki/Internet_protocol_suite check this out.