Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial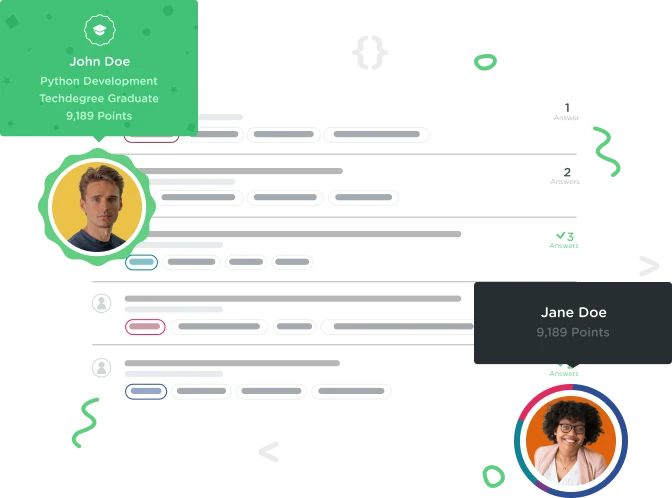
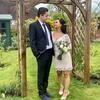
Gavin Johnston
9,107 PointsHow does the setTimeout function knows that the argument should be passed to the function expression?
I really dont understand this.
window.setTimout((something) => {
console.log(something);
}, 3000, 'Hello');
The string hello is not part of the anonymus function which acctepts an argument for (somthing), yet it is passing the string Hello to it.
Any help appreciated, very confused here.
3 Answers
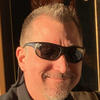
Peter Vann
36,427 PointsHi Gavin!
I can't exactly tell you why or how it works (I'd have to see the actual setTimeout source code), but just that it does work that way - and for multiple parameters.
(setInterval is similar, too)
Like This:
<!DOCTYPE html>
<html>
<body>
<p>Click "Try it". Wait 3 seconds, and the page will alert "Hello".</p>
<button onclick="setTimeout(myFunction, 3000, 3, 5);">Try it</button>
<script>
function myFunction(num1, num2) {
alert(num1 * num2);
}
</script>
</body>
</html>
Will produce an alert box with 15 in it.
setInterval must be coded something like this, though:
const setTimeout = (callbackFunction, delayInterval, ...args) => {
// some code that waits/sleeps for the duration of the delayInterval in miliseconds
callbackfunction(arguments); // call the callback function after waiting and pass in the exploded argArray
}
More info:
https://programming.vip/docs/source-code-analysis-of-settimeout-and-setinterval.html (Didn't quite grasp this, to be honest!?!)
https://usefulangle.com/post/198/javascript-settimeout-pass-parameter
I hope that helps.
Stay safe and happy coding!
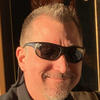
Peter Vann
36,427 PointsI sort of added my idea of how setTimeout is probably wired-up (it's not perfect, but it should help you visualize the "guts" of the function, theoretically, anyway)!?!
(Look at the middle of the original post.)
I hope that helps.
Stay safe and happy coding!
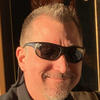
Peter Vann
36,427 PointsThis might help, too:
const square = function(num) {
return num * num;
}
const quad = function(func, num) { // Notice that func is a callback function
return func(num) * func(num);
}
console.log( quad(square, 2) ); // Which becomes square
Which will log 16.
Or then you could also do this:
const cube = function(num) {
return num * num * num;
}
const quad = function(func, num) { // Notice that func is a callback function
return func(num) * func(num);
}
console.log( quad(cube, 2) ); // Which becomes cube, in this case
Which will log 64
I hope that helps.
Stay safe and happy coding!
Gavin Johnston
9,107 PointsGavin Johnston
9,107 PointsIt seems to just pass the arguments based on the order they appear without having to specifically tell it to do so, for instance..
Would output..
1 2 3 2 1