Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial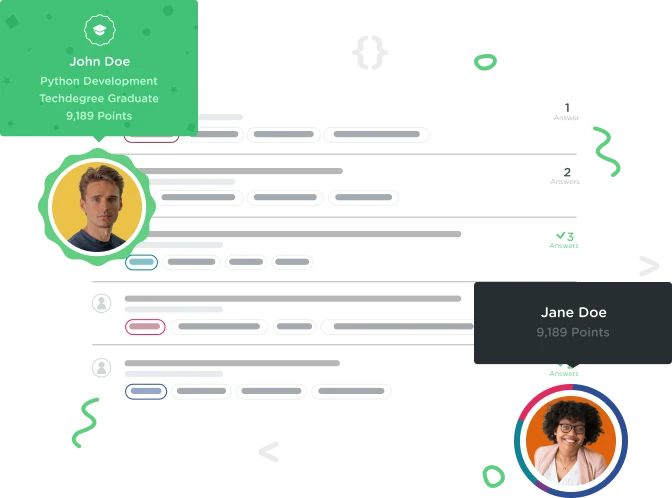

Jerry Liu
Courses Plus Student 1,233 PointsHow does the sort compare function work?
In the code snippet below:
var my_array =[566, 3, 9, 24, 1];
my_array.sort(function(a, b){
return 1;
});
console.log(my_array);
the compare function will reverse the order of a and b, but It only reverse two elements at a time. How does this reversion apply to the whole array to deliver the final result?
2 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsThe JavaScript standard only defines the behaviour of the compare function, not how the actual sort is implemented. That parts depends on the vendor/environment.
But the basic idea is: if every element in the array is compared with all the other elements in the array, the array gets sorted. There are of course shortcuts that the actual algorithm takes to speed up the process and optimise it in general.
So, the compare function gets called numerous times, until all combinations of a
and b
are exhausted from the elements in the array.

Jason Anello
Courses Plus Student 94,610 PointsHi Jerry,
As Dino mentioned, the sort method is going to use a particular sorting algorithm to get the entire array sorted. Which algorithm used is implementation dependent.
You may want to pick one of the simpler sorting algorithms and read the high level description of it and try to sort your example array either in your head or on paper. You can do this without getting involved in the code just to understand it at a higher level.
Was your goal here to reverse the array?
One thing that I would like to point out is that I think this is only going to work out correctly if the sort is stable. See the "stability" section in the wikipedia article for the difference between stable and unstable. In particular, the card diagram makes it easy to see the difference.
For example, the following array var my_array =[1,2,3,4,5,6,7,8,9,10];
will be correctly reversed in both firefox and chrome using your compare function.
Add one more element to the array var my_array =[1,2,3,4,5,6,7,8,9,10,11];
and firefox will still reverse it correctly but chrome will not.
I believe that firefox implements a stable sort across the board, I'm only guessing here. Chrome uses a stable sort for up to 10 elements but then switches to an unstable sort for more than 10.
I know this wasn't the point of your question but this is just something to be aware of. You can't necessarily achieve any kind of sorting you want.
A more reliable way to reverse an array would be to use the reverse
method or if you wanted to implement yourself then you could swap the first element with the last, the 2nd with the 2nd to last, and keep working towards the center.