Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial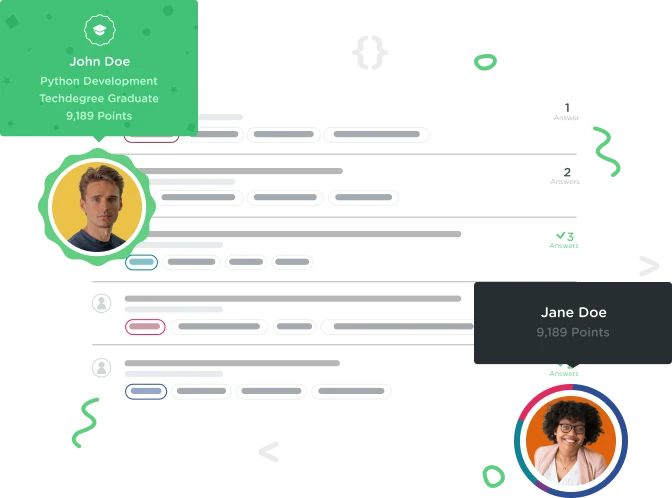

Cassie Wilson
1,827 PointsHow does this block of code stop a repeating guess?
I don't understand how this code keeps guesses from repeating themselves:
if(mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) { throw IllegalArgumentExcepetion (letter + " has already been guessed."); }
I do understand why you include both the Misses and the Hits, but I guess I don't know how it knows what letter the guesser guesses.
Thanks for the help!
1 Answer

lambda
12,556 PointsNext time please include the video or exercise you were looking at so that it can provide more context to your problem.
But from your code snippet:
if(mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw IllegalArgumentExcepetion (letter + " has already been guessed.");
}
can be shortened to:
if( (any number bigger than 0) >= 0 || (any number bigger than 0) >= 0 ){
throw IllegalArgumentExcepetion (letter + " has already been guessed.");
// this will trigger the exception and stop the program
}
So if the letter guessed is in either mMIsses or mHits, it will return a number than is greater than or equal to 0 which makes the if condition true triggering the exception will stop the execution of the program.
It looks like mMisses is the string containing all letters that were guessed but incorrect and mHits is the string containing all letters that were guessed and were correct.
For Example:
when you start the game (no guess made yet):
_ _ _ _ _ _ _ _ _ _ _ (mAnswer = "programming" but letters are hidden with "_")
mMisses = "" (no wrongly guessed letters yet)
mHits = "" (no correctly guess letters yet)
after you have made some guesses:
p r _ g r _ _ _ i _ g (mAnswer = "programming", the letter guessed correctly are shown)
mMisses = "aeuyzx" (all the letter you guess that were incorrect)
mHits = "prgi" (all the letters you guessed that were correct)
the strings mMisses and mHits will grow longer as you continue to play hangman.
If you look at the documentation link for the indexOf() method for Strings:
indexOf(String str)
Returns the index within this string of the first occurrence of the specified character.
Cassie Wilson
1,827 PointsCassie Wilson
1,827 PointsSorry, this was my first time using the forum. I didn't realize it didn't automatically link with the video. It was the Java Objects: Validation video.
And yes, earlier we did define the member variables mHit and mMisses, but we left them open. It looks like this:
public class Game { public static final int MAX_MISSES = 7; private String mAnswer; private String mHits; private String mMisses;
public Game(String answer) { mAnswer = answer; mHits = ""; mMisses = ""; }
So when you leave it undefined with "", does that later store the guesses you put in?
Thank you so much for your help.
lambda
12,556 Pointslambda
12,556 PointsSo when you leave it undefined with "", does that later store the guesses you put in?
as we play the hangman game, we are guessing letters and the wrong letters are added to the mMisses string, and the correct letters are added to the mHits string.
For Example:
Also, I forgot to mention that the if-statement is probably inside a loop of some sort. And the if-statement loops and loops until it is kicked out of the loop by the exception.
Cassie Wilson
1,827 PointsCassie Wilson
1,827 PointsYou explained it perfectly. I completely understand it now.
Thank you!
lambda
12,556 Pointslambda
12,556 PointsYou're welcome. If you could click "Best Answer" next to "Add Comment" so that (in the future) if someone comes across the same question, they can just read this explanation instead of re-asking the same question.