Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial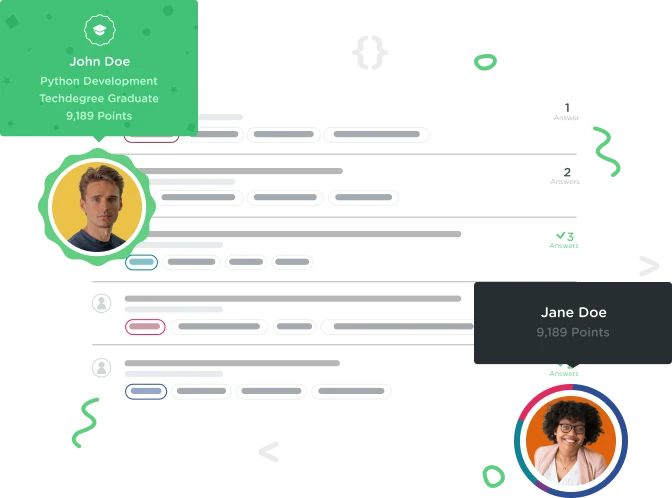

Enzo Cnop
5,157 PointsHow does this code check if the number is even/odd?
I passed the challenge my copy and pasting from another help thread. I don't understand how this concept works. How does the 'even_odd' function check against the randint? I see the 'if even_odd(randint):' line, but how does this decide if it is true or not against 5? Also, the 'start -= 1' line seems to be part of the 'else' statement. How does it run if the first function goes through?
import random
start = 5
def even_odd(num):
return not num % 2
while start:
randint = random.randint(1, 99)
if even_odd(randint):
print("{} is even".format(randint))
else:
print("{} is odd".format(randint))
start -= 1
Why can't the code read
import random
start = 5
def even_odd(num):
return not num % 2
while start is True:
num = random.randint(1, 99)
even_odd(num)
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
I'm guessing this doesn't work because start is never modified, and as a result it will always = 5, meaning it will always be True?
1 Answer
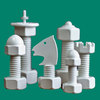
Steven Parker
229,732 PointsThe modulus operator is used to determine even/odd status.
That's what "num % 2
" is doing. The modulus, or remainder, will be 1 for odd numbers and 0 for even numbers. Then the "not
" operator is used to flip that to make the result True for even numbers and False for odd.
And "start -= 1
" is not part of the else statement because it is not indented far enough. Anything part of the else would have to be indented more than the else itself.
Enzo Cnop
5,157 PointsEnzo Cnop
5,157 PointsThank you for your reply. I understand now what is happening with the 'not num % 2' -- I still would like to know why start = 5. Could start = True in this situation and produce the same result?
Steven Parker
229,732 PointsSteven Parker
229,732 PointsSince start is being used as a counter, it needs to be number. Each time through the loop, the value is reduced by one. When it reaches 0, the loop stops running.
Enzo Cnop
5,157 PointsEnzo Cnop
5,157 PointsAha! Now I understand what is happening. The code takes a random number and checks if it is even or odd 5 times before breaking. If the challenge had asked us to do this in the first place, I think I would have been less confused. Thank you again!