Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial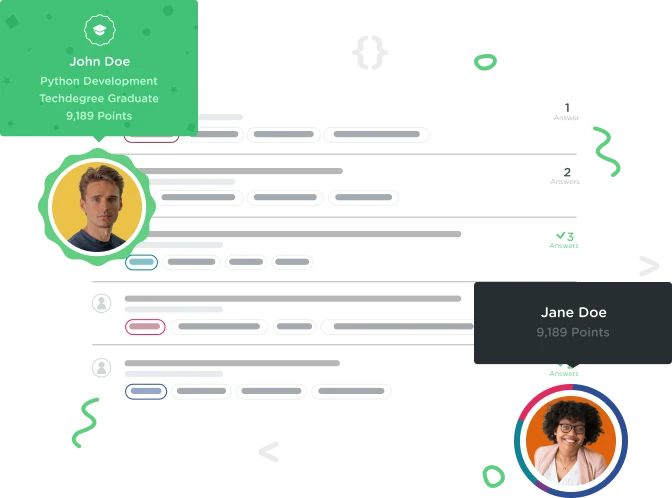

Venera Dev
1,747 PointsHow does this compare is work? Step by step. What is mean " return 1" "return -1"? How "return" value affect for sort ?
var items = ['4' , '2' , 'zo', 'bo', '1' ,'Ams', 'B', 'a', 'A'];
items.sort(function(a,b) {
if (a < b){
return -1;
}
if (a > b){
return 1;
}
return 0; });
// --> [ '1', '2', '4', 'A', 'Ams', 'B', 'a', 'bo', 'zo' ]
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Venera,
The sort method can optionally take a compare function as an argument if you need a sort order that's different from the default sort order which is to sort based on Unicode code points. This means a character with a lower code point will come before one with a higher code point. This is how your example is sorting so you don't technically need the compare function here.
If you need something different from this then you need a compare function.
The idea here is that the sort method will pass 2 elements at a time to the compare function and the job of the compare function is to return a value to indicate what order the 2 elements should be in.
If a negative value is returned, doesn't have to be -1, then 'a' should come before 'b'
If a positive value is returned, doesn't have to be 1, then 'b' should come before 'a'
If zero is returned then the 2 elements are considered equal.
Let's say that you wanted to sort those strings based on their lengths, shortest strings first. Now you would need a compare function because the sort method does not know how to do this.
Using your array example, suppose that the sort method passes 'zo' for a
and 'Ams' for b
. 'zo' is the shorter string so that means that a
should come before b
in this case. That means that our compare function better return a negative value to indicate that. If the sort method gets back a negative value then it knows it needs to make sure that 'zo' comes before 'Ams' in the final sorted array.
The compare function would look like this:
function(a,b) {
if (a.length < b.length) {
return -1;
}
if (a.length > b.length) {
return 1;
}
return 0; }
It can be shortened down to this,
function(a, b) {
return a.length - b.length;
}
Here's some output to show that they're sorted by string length in increasing order:
> var items = ['4' , '2' , 'zo', 'bo', '1' ,'Ams', 'B', 'a', 'A'];
undefined
> items.sort(function(a, b) { return a.length - b.length })
[ '4', '2', '1', 'B', 'a', 'A', 'zo', 'bo', 'Ams' ]
>
Here's the mdn page for the sort method if you want to know more details: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/sort
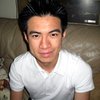
jason chan
31,009 Pointsvar a = [2, 9, 9];
a.indexOf(2); // 0
a.indexOf(7); // -1
if (a.indexOf(7) === -1) {
// element doesn't exist in array
}
-1 means the item doesn't exist in the array. That's what that means.
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/indexOf
You can read more about this in the documentation.