Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial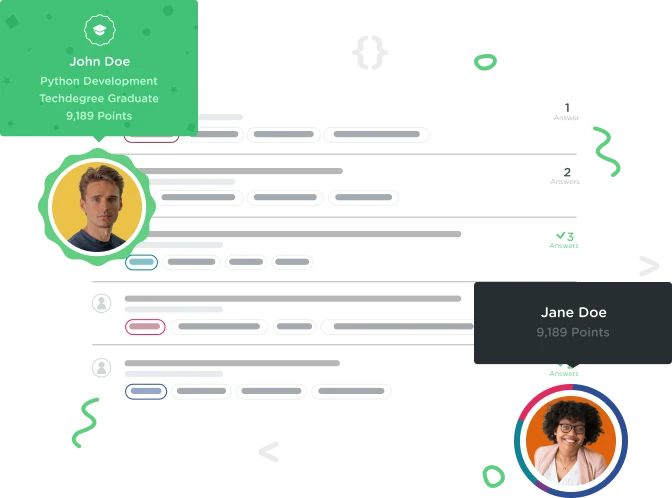
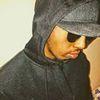
ibrahim Warsame
7,803 PointsHow does this program work?
I'm really confused :(
import java.io.*;
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter prompter = new Prompter(game);
boolean isHit = prompter.promptForGuess();
if(isHit) {
System.out.println("We got a hit");
}else {
System.out.println("Whoops we missed that one");
}
}
}
can anyone explain to me how the code above works in great details, especially this part:
boolean isHit = prompter.promptForGuess();
I'm new to java programming, not necessarily programming. Java feels weird to me but i'm beginning to like it :)
5 Answers

Aaron Arkie
5,345 PointsAt the beginning of the implementation of the main method we have a Game object being created. This game object has an argument of type String because it has a parametrized constructer. Constructors are automatically called when a new object is created. If you did not create a constructor the compiler will automatically generate one for you called a default constructor which takes no arguments. Generally, constructors are great at initializing member variables or starting an important process.
Next we have another object being created but this is of type Prompter that also has a paramterized constructor that takes an object as its argument.
The next line over declares a Boolean variable that calls the method promptForGuess from the Prompter object. This method will return a value of TRUE or FALSE to the calling object and store it in the isHit variable. I'm guessing the method promptForGuess asks the user for input. Once the input is read it compares it to the Keyword you are trying to guess correctly. If the guess was correct it will return TRUE or if not it will return FALSE.
Which leads us to our if-else statement. If isHit = True then print out to stdio that you got a hit else if its false print out to stdio that you missed.
if(isHit)
{
System.out.println("We got a hit");
}
else
{
System.out.println("Whoops we missed that one");
}
// is the same as
if(true == isHit)
{
System.out.println("We got a hit");
}
else
{
System.out.println("Whoops we missed that one");
}
I hope this helps! You said you know some programming so if you need me to go deeper into a particular part let me know.
Keywords & phrases:
Object, Constructors, calling a method, return type

Aaron Arkie
5,345 PointsHello Ibrahim,
The readLine method is being called in the Prompter class.
// Stores user input in String variable called guessAsString
String guessAsString = console.readLine("Enter your guess: ");
// Gets the first character of the guessAsString variable and stores it in the variable called guess
char guess = guessAsString.charAt(0);
So once we call prompter.promptForGuess() we run this line of code to get the users input from the keyboard.
I would need to see the Game class because this line of code
return mGame.applyGuess(guess);
It goes into an object of the Game class and uses the method applyGuess(char guess) which more than likely returns TRUE or FALSE which is then stored in the isHit variable.
You could also break it up one step further to see what's going on.
import java.io.Console;
public class Prompter
{
private Game mGame;
public Prompter(Game game)
{
mGame = game;
}
public boolean promptForGuess()
{
Console console = System.console();
String guessAsString = console.readLine("Enter your guess: ");
char guess = guessAsString.charAt(0);
boolean checkGuess = mGame.applyGuess(guess);
return checkGuess;
}
}
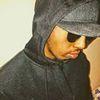
ibrahim Warsame
7,803 PointsThanks for the help :)

Aaron Arkie
5,345 PointsNo problem good luck in your studies!
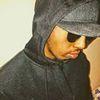
ibrahim Warsame
7,803 PointsYou are really good at this. When did you start.

Aaron Arkie
5,345 PointsI started to learn a little bit by myself when I was 18( now almost 22). Java was my first language! I started off with the New Boston tutorials on youtube and then bought myself a book to learn the language. After some practice I switched over from an Arts major to a Computer Science major.
I recently just took a class last semester which was an intro class to computer science using C++. My professor was amazing, he worked at Symantec building software for Norton Antivirus, and he is so passionate about programming. I mean this guy really knew his stuff! So now his practices and enthusiasm is something I hope I can achieve and now I'm just immersing myself into programming.
If you ever can, I highly recommend taking a course on C++. You'll learn how programming works with memory(RAM) and it will demystify all of the processes that occur behind the scenes. It's definitely more hands on and doesn't protect you like Java does. It's also, in my opinion, a beautiful language.

Aaron Arkie
5,345 PointsI'm really not that good. I still have a lot to learn, however, practice is key to everything. By yourself a book and go through every practice problem. By a whiteboard and start creating flowcharts. The more you practice the more you'll be comfortable with solving problems with programming. Once you learn one language you should be able to transition to other languages more easily because they are almost all the same. Syntax is the only thing that changes.
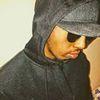
ibrahim Warsame
7,803 PointsWell, thanks man. I'm really looking forward to beginning university. Right now I'm 15 and I really like programming, i stopped playing games(Minecraft, league of legends, counter strike global offensive or CS: GO for short and so on) in order to make cool things with java. I hope that pays off. and thanks for replying :)

Aaron Arkie
5,345 PointsForsure just keep at it! Make it a part of your everyday life and by the time you start college you'll be way ahead of your colleagues! Dont stop learning and have fun with it.
Also, you can learn game programming if thats where your interests are. You can learn java and build small applets and switch over to android. Or learn a game engine such as unity or unreal.
Good luck!
ibrahim Warsame
7,803 Pointsibrahim Warsame
7,803 PointsThanks for the help, but i dont really understand how is the isHit variable gets input from the user, dont we need console.readline() in order to get input from the user. Let me show you the prompter class file in case you need it:
I thought that if we do something like this:
getsInput = console.readline("What is your name: ")
That we need to do something like this in order to get the user input and store it in the getsInput so letter on we can use the value for what we want:
System.out.println(getsInput);
how is the isHit boolean variable getting an input from the user then, it dosent even have console.readLine()
thanks for advance :)