Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial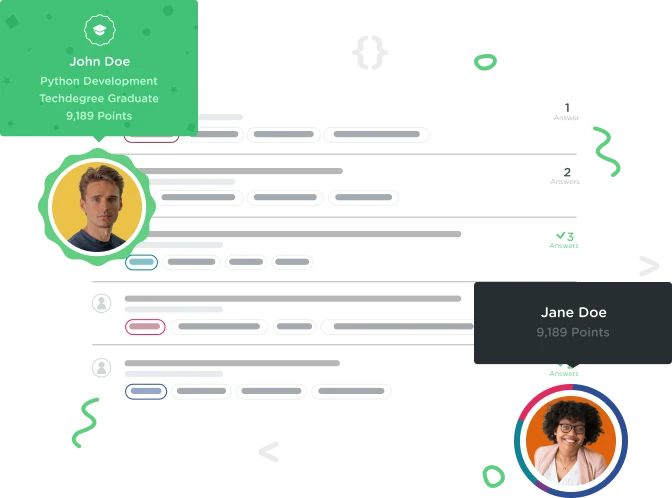
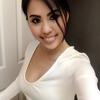
Vy Nguyen
4,350 PointsHow does this work?
:(
var money = 9;
var today = 'Friday'
if ( money < 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money < 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money < 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( money >= 8 || today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
1 Answer
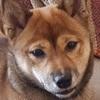
Katie Wood
19,141 PointsHey there,
There are two main steps to fixing this one - first, it's using OR (||) logic in all of the conditionals. This is a problem because one of the conditions is "today === 'Friday'" - since today is set to Friday at the top, it will always pass the first condition, and not go any further. We want to use AND (&&) logic instead, to check for the right amount of money AND the day for each condition. The other issue in the initial script it gives you is that the last 'else if' should have === instead of !== - it looks like you already caught that one! That condition doesn't need to check for money, though - since it will only get that far if none of the other conditions above it pass, that last one already implies that the money value is 10 or below.
Changing all of the or logic to and logic and changing that last else if's sign makes it look something like this:
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Hope this helps!