Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial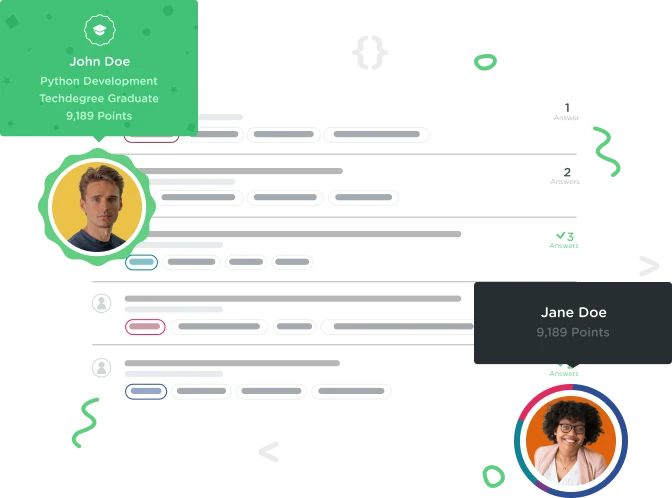
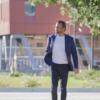
Bappy Golder
13,449 PointsHow does $(this) work in this case?
I'm just wondering how exactly $(this) work for the following instance?
$(document).ready(function(){
$('button').click(function(){
$('button').removeClass("selected");
$(this).addClass("selected");
});
}); // end ready
Dave said that the $this
will only select the button clicked. But the $('button')
is selecting all the buttons. How does $this
knows which button is selected?
2 Answers
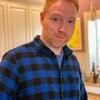
Jason Arnold
22,110 PointsLaVaughn has it right. 'This' references the object that started the action. In this case it is the particular 'button' that is clicked to invoke the click event handler.
this is the handler for the click event for any button. When ANY button is clicked do what is inside this function.
$('button').click(function(){
removes the "selected" CSS class from ALL buttons when ANY button is clicked
$('button').removeClass("selected");
adds the "selected" CSS class back to the button that was clicked
$(this).addClass("selected");

LaVaughn Haynes
12,397 Points"this" can be a difficult thing to understand in JavaScript because it can mean different things in different situations.
In your example, "this" refers to the button that was clicked. When you use the jQuery selector it's like executing a search. So $('button') means "look at my document and give me all of the buttons". $(this) means "give me the button that was clicked"
$(document).ready(function(){
$('button').click(function(){
//removes the selected class from ALL of the buttons
//ALL BUTTONS
$('button').removeClass("selected");
//adds the selected class to the button that was clicked
//JUST THIS BUTTON
$(this).addClass("selected");
});
}); // end ready

LaVaughn Haynes
12,397 PointsAlso, when the button is clicked it passes a whole bunch information to your function about the event and the element that was clicked. If you open your console and click a button while using this code you can see the object.
$('button').click(function(e){
console.log(e);
});