Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial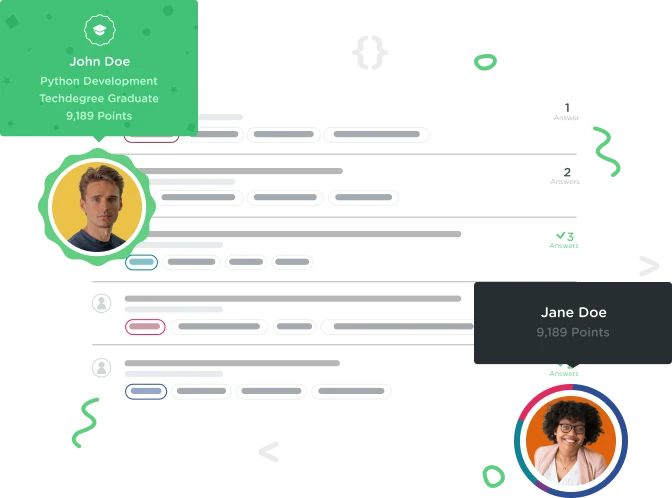

nicholasdevereaux
16,353 PointsHow does throwing an error affect the user?
I'm not fully understanding why we throw the error just to print it to the console? How would the programmer use that information? And how would the error affect the user? Would we display an alert message to let them know they need to use a number?
1 Answer

Lars Reimann
11,816 PointsIn general, you want to separate the normal control flow of your program from the error control flow. C, another programming language, does not have specials constructs to throw and handle errors. Instead you use the return value of the function to indicate if anything broke. In C you can only return one value, just like in JavaScript, so you need to pass the actual result back to the caller in another way. I won't go into the details, but the code is not particularly intuitive compared to most other programming languages. You are basically using parameters to pass back results.
Say you want to open a file, read it's content and write it to the console. The code could look something like this in JavaScript if we had appropriate functions and those output parameters from C:
var file;
var content;
var error = openFile('path', file); // file is the output parameter; if the function call succeeded, we can read from it
if (error !== 0) { // a non-zero value indicates problems
handleError(error );
} else { // only continue if we opened the file
error = readFile(file, content) // content is the output parameter
if (error !== 0) {
handleError(error);
} else {
console.log(content);
}
}
So you end up with some bloated, potentially nested code and a lot of repetition (ifs and handleError after every step). And this is just a simple example. Imagine if we wanted to open a second file and write the content of the first one there. What we really want here is to stop executing the remaining steps when something goes wrong. And this is where exceptions are great. Suppose the openFile functions and the like would throw an exception if something goes wrong. First of all, this frees up the return value for the value we really want to return, so we can get rid of this weird output parameter stuff. But using another JavaScript control statement, namely try-catch, we can catch any exceptions that are thrown in the try-block and execute the code in the catch-block. This is best seen in an example, so let's modify the above program:
try {
var file = openFile('path');
var content = readFile(file);
console.log(content);
catch (error) {
handleError(error);
}
Much nicer, right? If something goes wrong, when we open the file, we will handle the error in the catch-block and we won't execute the rest of the try-block. I. e. we will never read from the file or print its content.
As an added benefit, a thrown error is not just a number like in C. So there is no need to look up what an errorcode of 42 means. You can set a helpful message and browsers automatically create a stacktrace that tells you where the error occurred in your program and more.
To sum things up for your question: You usually try to handle an error using try-catch, so the user won't even notice that something went wrong unless you specifically tell them. Like you said, in the random number example, a reasonable course of action would be to display a message to the user in the catch-block. If some error occurs that is not caught explicitly by you, the program will terminate. This is still better than if it would try to keep running in some corrupted state.