Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial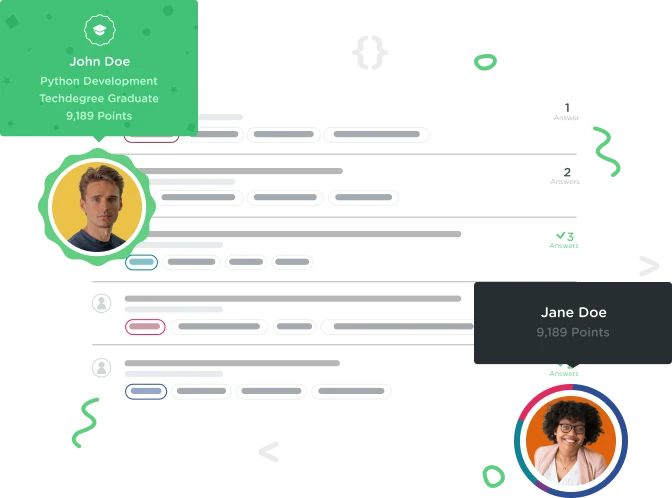

Iliya Narsiya
2,054 PointsHow enumerate properties the object in which have another one object?
How enumerate properties the object in which have another one object? Please, with comment on code.
For example:
var person = {
name:"Sarah", age: 35, country: "USA",
size: { top: 90, middle: 60, bottom: 90 }
}
2 Answers
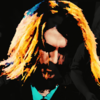
Justin Horner
Treehouse Guest TeacherHello Iliya,
In order to loop through inner objects you would use a for...in loop on the property of the object like this.
var person = {
name:"Sarah", age: 35, country: "USA",
size:
{
top: 90,
middle: 60,
bottom: 90
}
}
for (var key in person.size) {
console.log('Key: ', key, ' Value: ', person.size[key]);
}
I hope this helps.
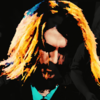
Justin Horner
Treehouse Guest TeacherHello Iliya,
Sorry for the misunderstanding. If you want to loop through every key value pair in an object, you can use the typeof operator to discover which properties are objects. Once you know that, you can use the function recursively to print the properties of the inner object and so forth.
Here's an example of how you could accomplish this with your object example.
function printObjectDetails(obj) {
for (var key in obj) {
console.log('Key: ', key, ' Value: ', obj[key]);
if (typeof obj[key] === 'object') {
printObjectDetails(obj[key]);
}
}
}
var person = {
name:"Sarah",
age: 35,
country: "USA",
size:
{
top: 90,
middle: 60,
bottom: 90
}
}
printObjectDetails(person);
I hope this helps.

Iliya Narsiya
2,054 PointsThank you, for you try help me!
I confused, that function print out - Value: [object Object] instead - size Key: top Value: 90Key: middle Value: 60Key: bottom Value: 90
Key: name Value: SarahKey: age Value: 35Key: country Value: USAKey: size Value: [object Object]Key: top Value: 90Key: middle Value: 60Key: bottom Value: 90
Iliya Narsiya
2,054 PointsIliya Narsiya
2,054 Pointsvar fruits = { 'apple': { 'color': 'green', 'sort': 'Granny Smith', 'Country': 'Russia', 'price': 68 }, 'orange': { 'color': 'orange', 'sort': 'Valencia', 'country': 'Spain', 'price': 100.99 }, 'pear': { 'color': 'red', 'sort': 'Conference', 'country': 'Cyprus', 'price': 120.59 }, 'netto' : 125600 // not is object };
I was meant, how print out all properties, that be to look like :
apple : color = green apple : sort = Granny Smith apple : Country = Russia apple : price = 68 orange : color = orange orange : sort = Valencia orange : country = Spain orange : price = 100.99 pear : color = red pear : sort = Conference pear : country = Cyprus pear : price = 120.59 netto = 125600