Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial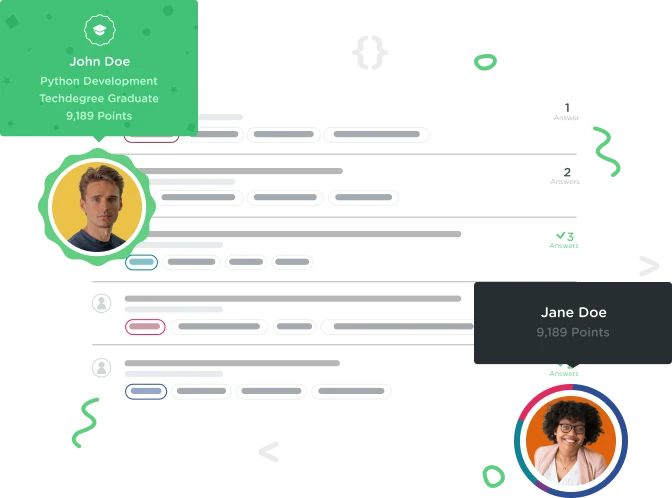

ShaoHsuan Duan
6,044 PointsHow exactly do try and catch work?
Hello, I'm really confused about how try and catch actually work and am trying to clarify my mind in this question.
Here is the part of "try" and "catch"
try{
isHit = mGame.applyGuess(guess);
isValidGuess = true;
}
catch (IllegalArgumentException iae){
console.printf("%sPlease try again.\n", iae.getMessage());
}
And here is the part of "throw new exception" along with the "applyGuess" method
private char validLetter(char letter){
if(!Character.isLetter(letter)){
throw new IllegalArgumentException("A letter is required\n");
}
if(mHits.indexOf(letter)>=0 || mMisses.indexOf(letter)>=0){
throw new IllegalArgumentException("You have tried this letter brfore.\n");}
letter = Character.toLowerCase(letter);
return letter;
}
public boolean applyGuess(char letter) //see if the letter hit
{
letter = validLetter(letter);
boolean isHit = mAnswer.indexOf(letter)>=0; //if mAnswer contains the letter the player entered. if yes, you'll get the index of the letter, if no, you'll get -1
if(isHit){
System.out.printf("Hit!\n");
mHits += letter;
}
else if(!isHit){
System.out.printf("Miss!\n");
mMisses += letter;
}
return isHit;
}
My question is, if the exceptions in "validLetter()" happen, does "letter = validLetter(letter);" break the "applyGuess()" method?
And does this result the inability of executing this line "isHit = mGame.applyGuess(guess);" and break the "try block" and so it won't continue execute the next line which is "isValidGuess = true;" ?
2 Answers

miguelcastro2
Courses Plus Student 6,573 PointsWhen the applyGuess() method runs it will immediately call the validLetter() method to check if the letter is a valid character. If not, validLetter() will throw an exception. The exception is triggered on the line when applyGuess() is called:
isHit = mGame.applyGuess(guess);
When the exception is thrown, the code will stop processing and immediately jump to the catch{} block. The line:
isValidGuess = true;
Will never run.

miguelcastro2
Courses Plus Student 6,573 PointsOne last thing, the applyGuess() method must throw an IllegalArgumentException() if there is not try/catch block within the method:
public boolean applyGuess(char letter) throws IllegalArgumentException {}

Craig Dennis
Treehouse TeacherThis isn't required as that is a RuntimeException, but thanks for the good practice.