Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial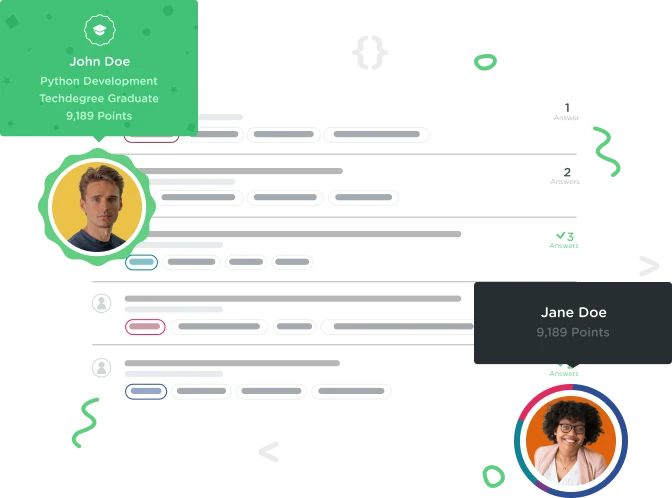
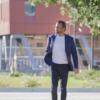
Bappy Golder
13,449 PointsHow is the for each parameter running?
I was looking at the MDN documentation for the forEach() method. And I tried the following code example:
//practice using foreach to loop over an array and show the values in the console
const arrA = [5, 14, 23, 32];
//write a function to displey the elements of the array
logArrayElement = (element, index, array) => {
console.log('arrA[' + index + ']' + ' = ' + element);
};
arrA.forEach(logArrayElement);
- What I don't understand is how the last line of code is working. It seems we can pass in a function inside a method. However we are not declaring any parameter. However the code runs just fine.
Can anyone please explain to me what exactly is happening here? Thanks a ton in advance.
1 Answer

andren
28,558 PointsThe logArrayElement function that is passed in to forEach does have parameters defined (element, index, array), as for passing in arguments to the function that is done by forEach itself. It takes whatever function you pass to it and calls it with three arguments, the first being the item it is currently on, the second being the index of the item and the third is the array it is actually pulling items from.
The code in your example is functionally identical to this code:
const arrA = [5, 14, 23, 32];
logArrayElement = (element, index, array) => {
console.log('arrA[' + index + ']' + ' = ' + element);
};
for (let i = 0, i < arrA.length; i++) {
logArrayElement(arrA[i], i, arrA)
}
The array is being looped over and the function that is passed in is just called with three parameters that the forEach function always passes along to the functions you pass it.
Bappy Golder
13,449 PointsBappy Golder
13,449 PointsThanks Andren,
I'm guessing that the
forEach
function knows about all those 3 aspects (array, index and currentPos) because we are running the code on the arrA (arrA.forEach(...
).If that is the case then it makes scense.
Thanks for the help.
andren
28,558 Pointsandren
28,558 PointsYes the forEach method has access to all three of those things. It has access to the array since it is being called on it, and since it is the one looping though the array it has access to the individual item and index, in the same way I have access to them in the for loop shown in my example.