Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial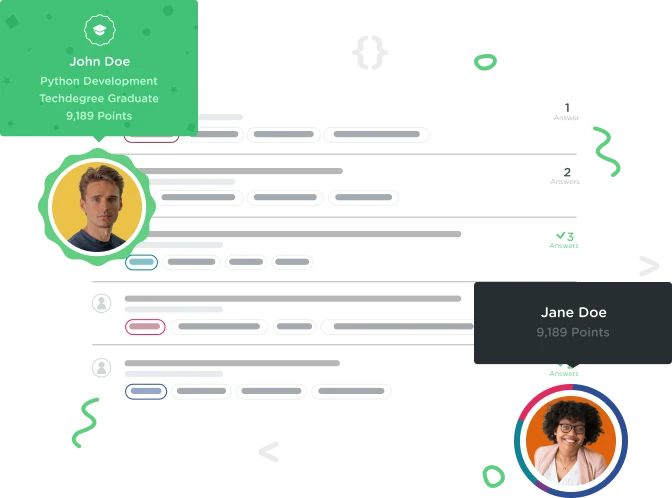

Sunny Chu
4,677 PointsHow is this not a true / false statement?
I am learning react and here is the code
function Application() {
return (
<div>
<h1>Hello from react</h1>
<p>I am render by reacttt</p>
</div>
)
}
My question is, how did the () mark after return is now acting as a DOM element, isn't it suppose to be a true or false statement?
And which plug in is making this happen? is it the JSX, Babel or react library?
3 Answers

Robert Adamson
5,741 PointsHi Sunny,
To start, the markup between the parentheses is JSX. Here is roughly how JSX becomes DOM elements on your page:
- Babel compiles JSX into 'react elements' using React.createElement
- ReactDOM transforms react elements and renders them in the DOM when appropriate
Return statements
You can return anything you want in Javascript. Any value is valid. Wrapping the return statement in parentheses is mostly a readability thing, ensuring that all of the code between them is returned. This is a very common practice in React because you are often returning JSX that is written on multiple lines. Additionally its a common practice in vanilla javascript to use parentheses any time you want to avoid 'automatic semicolon insertion' which is caused by terminating a line after the return keyword i.e. writing return statements which include line breaks.
In your specific example, the contents of the parentheses are actually all on one line. This would be a case where you do not need the parentheses and can simply return the JSX. I would assume the example you pulled this from is maintaining this as a convention of React returning JSX from component constructors.
Summary
- Your components return JSX, which Babel transforms into 'react elements', which ReactDOM uses to render in the DOM
- You can return any value in javascript, not just true or false
- Using parentheses is usually used as a way to allow the developer to add line breaks in their code while maintaining readability and full confidence in the entire block being returned. This is a convention used by most developers that use React
Recommended reading
- JSX and Babel: https://reactjs.org/docs/introducing-jsx.html
- React elements / ReactDOM: https://reactjs.org/docs/rendering-elements.html
- return syntax: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/return

Sunny Chu
4,677 PointsThanks alot Robert!!! This clears my question by a lot
Base on what you mention, can I interpret it as...?
- When I install Babel in my application, it will detect JSX file
- Banel will now compile the () within the JSX NOT as true or false, but as a DOM element into ReactDOM when I use it in conjunction with .createElement
- This causes a few issues, including : (1) I can't pass true or false, (2) I can't use a for loop

Robert Adamson
5,741 PointsYeah I'm glad I could help!
- Yes, typically when you install babel correctly it will understand how to transform JSX
- The short answer to your second question is 'basically yes'. A more precise answer is that babel will attempt to interpret and compile whatever you return from an expression as JSX, and transform it into a 'react element'. A react element is actually just a simple javascript object, it is not a DOM element. This can be very confusing at first, it confused me as well, but don't worry as it's not something you necessarily need to fully understand right away, as long as you're producing React components you will be able to pick it up eventually. The react elements are then used by ReactDOM to render the actual DOM element. I recommend you read this article (https://reactjs.org/docs/rendering-elements.html) as it explains further.
- You shouldn't pass true or false from a function that is attempting to construct a component. I'm not sure what react would do in this case actually, but I'm sure it would let you know that there was an error in compiling. I'm not sure exactly what you mean about using a for loop, but as long as you return valid JSX - which includes javascript in general - you can return it.
Good luck and have fun with React!