Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial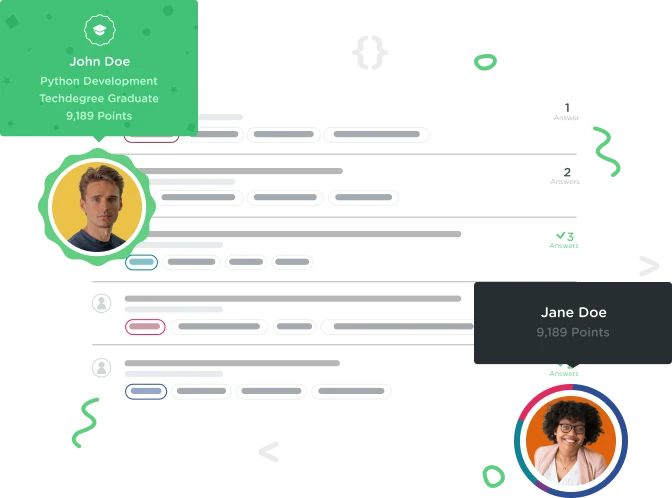

Rhew Deigl
1,047 PointsHow Is This Wrong?
When checked, the system tells me that it can't be compiled. I am wondering what I did wrong.
struct Expense {
var description = "String"
var amount = "Double"
}
var travel = Expense(description: "Flight to Cupertino", amount: 500.00)
2 Answers

Greg Kaleka
39,021 PointsHi Rhew,
In your struct definition, you are setting default values for the two properties of the string literals "String" and "Double". Note you're setting them to the strings "String" and "Double", not the data types String
and Double
. This would be just like setting them to "Potato" and "Monkey". Not only is this not what you're trying to do, but for this challenge, there's no need to set default values.
What you want to instead of assigning them values define their types. You do this with a colon, and since String and Double are keywords, there's no need for quotation marks (in fact you need to not use them).
struct Expense {
var description: String
var amount: Double
}
var travel = Expense(description: "Flight to Cupertino", amount: 500.00)
We're declaring the variables and just telling Swift what kind of variables they should be when initialized.
Let me know if this makes sense!
Best,
Greg
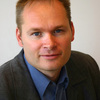
Knut Olaf Lien
7,743 PointsNote that you can set default values in a struct if this is what you want:
struct Expense {
var description = "Flight to Cupertino"
var amount = 500.0
}
var travel = Expense()
This will set the same values every time you declare an Expense variable. You can still override its values if you need.

Greg Kaleka
39,021 PointsThanks Knut - you're correct. I've updated my answer in light of this.
Knut Olaf Lien
7,743 PointsKnut Olaf Lien
7,743 PointsDid you mean to pass inn amount as String or as Double type? Try this:
Or this: