Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial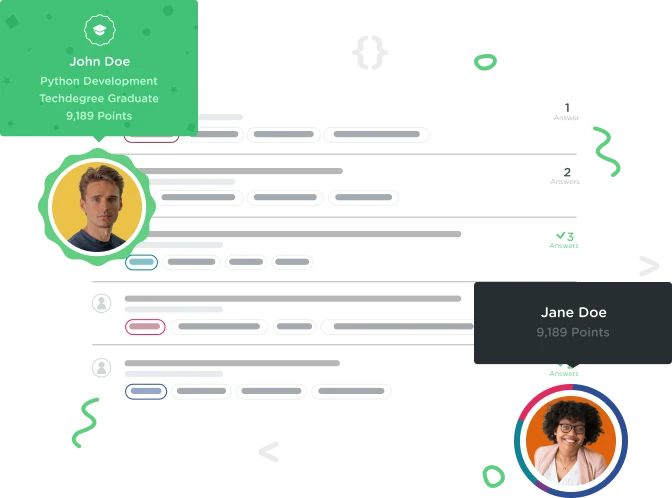

Napatchol Thaipanich
Courses Plus Student 13,534 Pointshow it do this challenge
In this challenge, we have the following geographical coordinates
Eiffel Tower - lat: 48.8582, lon: 2.2945 Great Pyramid - lat: 29.9792, lon: 31.1344 Sydney Opera House - lat: 33.8587, lon: 151.2140
Declare a function named coordinates that takes a single parameter of type String, with an external name for, a local name of location, and returns a tuple containing two Double values (Note: You do not have to name the return values). For example, if I use your function and pass in the string "Eiffel Tower" as an argument, I should get (48.8582, 2.2945) as the value. If a string is passed in that doesn’t match the set above, return (0,0)
// Enter your code below
func coordinates (for location : String) -> (lat : Double, lon : Double){
var lat = 0.0
var lon = 0.0
switch location{
case "Eiffel Tower" :
lat = 48.8582
lon = 2.2945
case "Great Pyramid" :
lat = 29.9792
lon = 31.1344
case "Sydney Opera House" :
lat = 33.8587
lon = 151.2140
default :
lat = 0.0
lon = 0.0
}
return (lat , lon)
}
var loc = coordinates (for : "Eiffel Tower")
loc.lat
loc.lon
5 Answers
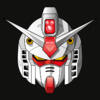
John Roque Jorillo
13,117 PointsIn the challenge it is mention that -> (Note: You do not have to name the return values) just remove the names of the return values.
// Enter your code below func coordinates (for location : String) -> (Double, Double){ var lat = 0.0 var lon = 0.0 switch location{ case "Eiffel Tower" : lat = 48.8582 lon = 2.2945 case "Great Pyramid" : lat = 29.9792 lon = 31.1344 case "Sydney Opera House" : lat = 33.8587 lon = 151.2140 default : lat = 0.0 lon = 0.0 } return (lat , lon) }
var loc = coordinates (for : "Eiffel Tower")
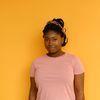
Marilyn Magnusen
14,084 PointsThis is just passed for me:
// Enter your code below
func coordinates (for location : String) -> (Double, Double) {
var lat = 0.0
var lon = 0.0
switch location{
case "Eiffel Tower" :
lat = 48.8582
lon = 2.2945
case "Great Pyramid" :
lat = 29.9792
lon = 31.1344
case "Sydney Opera House" :
lat = 33.8587
lon = 151.2140
default :
lat = 0.0
lon = 0.0 }
return (lat , lon) }
var loc = coordinates (for : "Eiffel Tower")
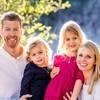
codyl
4,704 PointsThis seems cleaner (and just passed for me):
func coordinates(for location: String) -> (Double, Double) {
var lat = 0.0
var lon = 0.0
switch location {
case "Eiffel Tower": lat = 48.8582; lon = 2.2945
case "Great Pyramid": lat = 29.9792; lon = 31.1344
case "Sydney Opera House": lat = 33.8587; lon = 151.2140
default: lat = 0.0; lon = 0.0
}
return (lat, lon)
}

Arrington Copeland
23,505 PointsI did the same thing. I had to look up the semicolon also for this one.

Garrett Peters
6,039 PointsI do not recall semicolons being mentioned in the videos.

moonshine li
1,495 Pointsfunc coordinates(for location: String) -> (Double, Double) {
var lat: Double = 0.0
var lon: Double = 0.0
switch location {
case "Eiffel Tower":
(lat, lon) = (48.8582, 2.2945)
case "Great Pyramid":
(lat, lon) = (29.9792, 31.1344)
case "Sydney Opera House":
(lat, lon) = (33.8587, 151.2140)
default:
(lat, lon) = (0.0, 0.0)
}
return (lat, lon)
}