Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial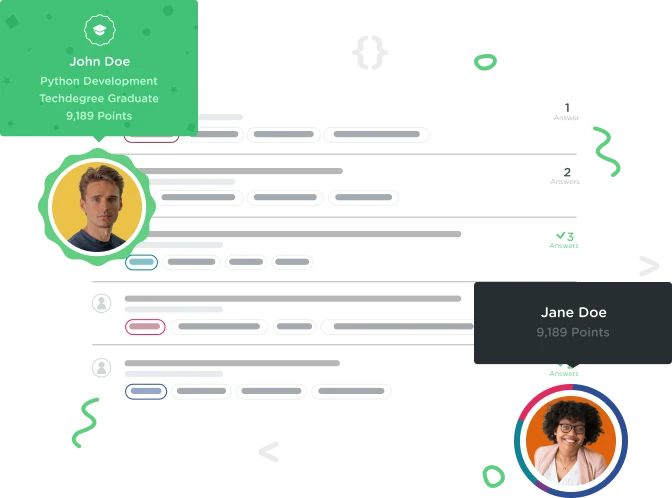

ammarkhan
Front End Web Development Techdegree Student 21,661 PointsHow key becomes a value
While studying objects, we use
for (var key in value){
console.log(key)
}
to access key of the object, but when we want to access its value we use
for (var key in value){
console.log(value[key])
}
I find it confusing that while we wanted to find out about only the keys, we used the first part key but when we wanted to find out its value, the same key is passed as a value.
1 Answer
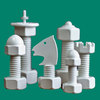
Steven Parker
231,169 PointsIt's not entirely correct to say "the same key is passed as a value." It would be more accurate to say "the same key is used as an index to access the value".
Another factor that might be making this particular example more confusing is the use of the word "value" to represent the object. So an example like this might make it more clear:
for (var key in object) {
var value = object[key];
console.log(`object.${key} = '${value}'`);
}
Brian Ball
23,661 PointsBrian Ball
23,661 Pointsyou'll need to translate code to words you understand. this could be more clear if written like:
for (var item in listOfItems) { console.log(listOfItems[item]) }
I translate this code as saying: for each key in the object(array) stored in a variable called value, console log the value of the item retrieved from the object named value referenced by value[key] <-- here, we're retrieving the value with the key (see how to reference an item in an object)
Imagine it looks like this value = { "key0" : value0, "key1" : value1 }
because you're using a for loop, the 0 and 1 are invisible as the loop is traversing each item