Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial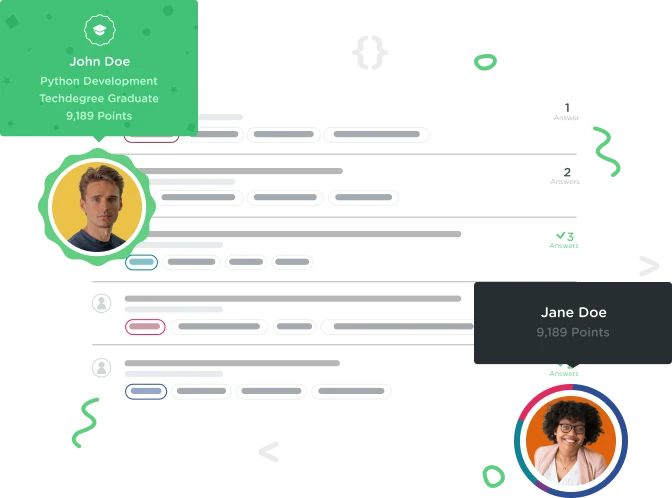

William J. Terrell
17,403 PointsHow might I use JavaScript or jQuery to compare a date?
On our website, we have an emergency alert system, which displays a banner at the top of the homepage in the event of severe weather, active-shooter, etc.
It works by looking at an RSS feed which, from what I can tell, is automatically generated by the local Emergency Alert System; if there is something in the feed, it displays it, but the feed has to be cleared out manually for the alert to go away.
The problem is that, the past couple weekends, we've had severe weather, but none of the people who maintain the feed were in to clear it, so we've had tornado alerts up on the homepage for days after the fact.
I would like to know if there is a way I can write some JS or jQuery to check to see if the content in the feed is more than a day old. If it is, I would like to set it to "not display".
To provide an example:
/* If I wanted to only display alerts that are
no older than 24 hours... */
if ( date != currentDate - 24 hours ) {
// do not display
} else {
// code to display alert banner
}
The only other thing is that I might end up having to figure out how to incorporate this into the Velocity (Java-based) code that is written for the alert system...
## change these variables to change the associated settings.
#set ($itemsToShow = 1) ## the total number of alerts to show.
#set ($messageLength = 140) ## the number of characters before truncating the message.
#set ($truncateString = "...") ## the string to display at the end of the message after it has been truncated.
##this is strange, but depending on where you test this, the xpath might be different. running some checks to change it based on the paths found so far.
#set ($items = $_XPathTool.selectNodes($contentRoot, 'channel/item'))
#if ($items.size() == 0)
#set ($items = $_XPathTool.selectNodes($contentRoot, 'rss/channel/item'))
#end
#if ($items.size() > 0)
#foreach ($item in $items)
#if ($velocityCount > $itemsToShow)
#break
#end
#set ($message = $_EscapeTool.xml($_DisplayTool.stripTags($item.getChild("title").value)))
#set ($message = $_DisplayTool.truncate($message, $messageLength, $truncateString, true))
<div class="alert top">
<div class="alert-container contained">
<p class="alert-message">
<span class="important-text">$_EscapeTool.xml($item.getChild("pubDate").value): </span>
$message
<a href="${item.getChild("link").value}">Read more.</a></p>
<span class="dismiss">Dismiss</span>
</div><!--alert-container contained-->
</div>
#end
#end
...which is still a bit above me at this point...
Nonetheless, any suggestions would be appreciated :)
Thanks!

William J. Terrell
17,403 PointsI don't know if it is the same as the actual emergency alert that goes out, but this is the formatting from the demo-feed:
<item>
<title>Email Into At 4:26</title>
<link>https://regroup.com/networks/demo/groups/123-regroup/topics/email-into-at-4-26</link>
<guid>https://regroup.com/networks/demo/groups/123-regroup/topics/email-into-at-4-26</guid>
<description><div dir="ltr">Email Into At 4:26<br></div></description>
<pubDate>Wed, 08 Jul 2015 18:26:50 CDT</pubDate>
</item>
2 Answers

William J. Terrell
17,403 PointsThanks for the feedback and information. As it turns out, I think I may have found a way to do it using the native Velocity code that most of the site runs on :)
Down in the Velocity Tools section on this page:
http://www.hannonhill.com/kb/Script-Formats/
it looks like it has a Comparison Date Tool built in.
Thanks just the same, though! :)

Iain Simmons
Treehouse Moderator 32,305 PointsMoment.js is a fantastic JavaScript library for dealing with dates and times. You can use that to 'parse' the dates coming in from the feed, and then compare to the current date.

Iain Simmons
Treehouse Moderator 32,305 PointsOh and if you're looking more generally into parsing RSS feeds in JavaScript/jQuery: How to parse a RSS feed using javascript? (StackOverflow)
Colin Marshall
32,861 PointsColin Marshall
32,861 PointsYou should be able to do this Javascript/jQuery. How is the date formatted in the feed? Can you link the live site so I can look at the code?