Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial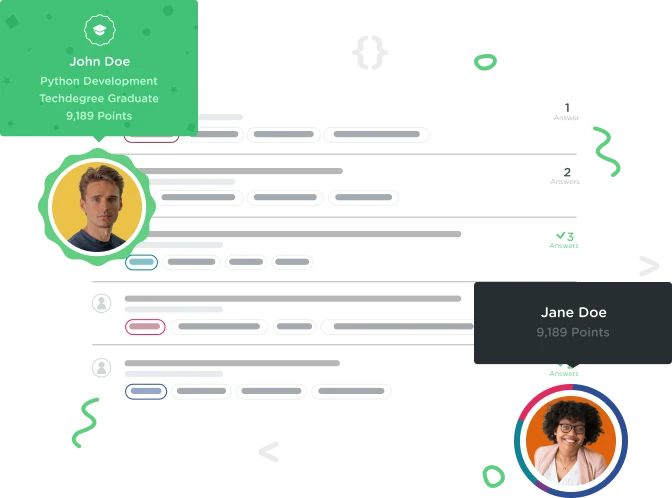
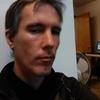
Mark Miller
45,831 PointsHow much more java do I need to import?
I am unsure of how to write this for-each-in loop with the Collection<E> type notation in the declarations for this method, to return a collection of all the BlogPost authors. I could be wrong about a small detail. Please help. It seems simple, but the Collection typing is confusing me.
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
Set<String> getAllAuthors(getPosts()) {
Set<String> allOurAuthors = new TreeSet<String>();
for(mAuthor: mPosts) {
allOurAuthors.addAll(mAuthor);
}
return allOurAuthors;
}
}
3 Answers
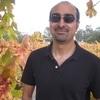
Kourosh Raeen
23,733 PointsHi Mark! A few points:
1) Mark the method as public.
2) The method does not need any parameters. All the posts are already in mPosts and available to the Blog class and any of its methods, including getAllAuthors().
3) Loop over all posts in mPosts. Note that I am calling the loop variable post and that I have say what type it is. In this case, it is of type BlogPost.
4) Then inside the loop call getAuthor() on each post and the returned author to your set using the add() method.
Try these and in case you're still stuck here's the complete code:
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> allOurAuthors = new TreeSet<String>();
for(BlogPost post: mPosts) {
allOurAuthors.add(post.getAuthor());
}
return allOurAuthors;
}
}
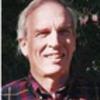
jcorum
71,830 PointsMark, it's not your imports. They look fine. You could do java.util.*; instead, although I know the instructor wants you to be explicit. The issues are in your method. Here's a suggested revision:
public Set<String> getAllAuthors() {
Set<String> allOurAuthors = new TreeSet<>();
for (BlogPost post : mPosts) {
allOurAuthors.add(post.getAuthor());
}
return allOurAuthors;
}
First, since this is a getter method you don't need a formal parameter. There's no need to pass any parameters into the method.
Second, you need to type the variable in the for each loop as BlogPost. I've changed the name of the variable from your mAuthor because each element is a post, not an author.
Third, you have to add Strings to your Set, not posts, so you need to use the getAuthor() method on each post.
Hope this helps.
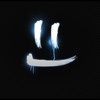
Grigorij Schleifer
10,365 PointsHi Mark,
you need to go over all posts in mPost and add the author of every BlogPost to the list that you have created.
So the for-loop should go like this:
Store every BlogPost from mPosts in "word" and extract the author from it. Then add the author to the TreeSet and return it.
The code could look like this:
public Set<String> getAllAuthors(){
// no parameter needed
Set<String> allOurAuthors = new TreeSet<String>();
for(BlogPost word: mPosts){
allOurAuthors.add(word.getAuthor());
}
return allOurAuthors;
}
Does it make sense?
Grigorij
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsCool answer, Kourosh !
Mark Miller
45,831 PointsMark Miller
45,831 PointsThank you for writing a clear, enumerated reply to my trouble. Now I can see. I understand that mPosts is already available without passing it in. And I realize that I should be looping through BlogPost objects in mPost. I also did not realize, yesterday, that I need to call getAuthor for each BlogPost object in mPosts. Now I can pass this quiz and move on to the remaining lessons in Java Data Structures. I've been stuck here for weeks.
Kourosh Raeen
23,733 PointsKourosh Raeen
23,733 PointsMark Miller - My pleasure! Glad you found the explanation helpful. Happy coding!