Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial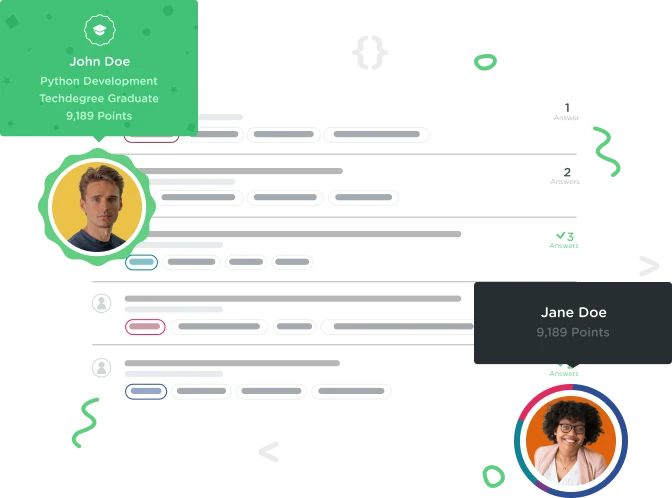

Jiho Song
16,469 PointsHOW SHOULD I DO? (JAVA)
I DONT KNOW HOW TO FIX IT
class GoKart {
private String color;
final Integer MAX_BARS;
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
}
1 Answer
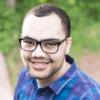
Philip Gales
15,193 PointsBummer! Make sure you've added a new int field using the proper naming style MAX_BARS
This error is telling us something is wrong with your variable 'MAX_BARS'. More specifically, it is saying you have not added the variable.
But you thought you already added it? Nope. Something is broken with your code.
There is a big difference between 'Integer' and 'int'. For variables, use 'int'. Let's do this and see what happens.
class GoKart {
private String color;
final int MAX_BARS; //<----------------notice I changed 'Integer' to 'int'
public GoKart(String color) {
this.color = color;
}
public String getColor() {
return color;
}
}
Now we have this error
Bummer! Make sure that you've made MAX_BARS public, we want people to use it!
This one is easy! Just declare the variable public.
public final int MAX_BARS;
Now we have
Bummer! Make sure that you've marked the MAX_BARS field with the static keyword. This will add the field to the GoKart class, for all GoKarts
Let's make the variable 'static'
public static final int MAX_BARS;
UGH! Another error.
Bummer! Make sure you initialize MAX_BARS to 8
public static final int MAX_BARS = 8;
Wow that was a lot of work, but we made it! Good job.