Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial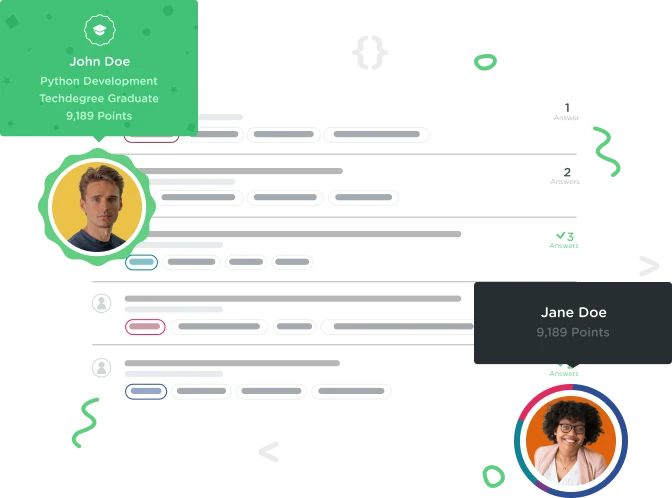

Clay Janson
96 Pointshow this code will work
please explain me
public void addSong(Song song){ mSongs.add(song); }
//this is main class
import com.teamtreehouse.model.Song; import com.teamtreehouse.model.songbook;
public class karaoke{
public static void main(String[] args){
Song Song = new Song(
"Justin bieber",
"Love Yourself",
"https://www.youtube.com/watch?v=oyEuk8j8imI"
);
songbook Songbook = new songbook();
System.out.printf("\nAdding %s\n",Song); // it will printout the toString method
Songbook.addSong(Song);
System.out.printf("There are %d number of song\n",Songbook.getSongCount());
}
}
Thank You!
3 Answers
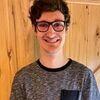
Trent Christofferson
18,846 PointsThe song variable is of type Song which contains the musician, song title, and link. You then create a songbook which stores all of your songs, like a karaoke machine stores songs. In the print statements %s will be replaced with what ever you put for example:
System.out.printf("My name is %s", string variable goes here);
the string variable will replace the %s in the string and the \n means what ever is after it will start on a new line. %d does the same thing as %s except it is used for numbers.

Nicolas Curat
2,995 PointsEverything Trent explained was perfect, but to add a few things regarding the lines: songbook Songbook = new songbook(); Songbook.addSong(Song);
By convention, in Java the first letter of the classes are written in capital letters and the variables are written in lower case characters, this is to distinguish the classes from the variables so i would recommend you to do it. For example, following what i just wrote, the line --> "songbook Songbook = new songbook();" should be "Songbook songbook = new Songbook();"
On the other hand, to answer your question, what the line "Songbook.addSong(Song);" does, is to call the method you wrote above that is in the class songbook: public void addSong(Song song){ mSongs.add(song); } and save the new song you created on the songbook.
Explained line by line:
public static void main(String[] args){
Song Song = new Song(
"Justin bieber",
"Love Yourself",
"https://www.youtube.com/watch?v=oyEuk8j8imI"
); //You create an instance of the class Song with the name of a musician, the name of a song and a link (all of which are String)
songbook Songbook = new songbook(); //You create an instance of songbook
System.out.printf("\nAdding %s\n",Song); // it will printout the toString method
Songbook.addSong(Song); //You take the instance of songbook and you add the new song you created before
System.out.printf("There are %d number of song\n",Songbook.getSongCount()); //You print the amount of songs that there are on the songbook (which in this case is only one).
}

Aditya verma
Courses Plus Student 664 PointsI am getting this error after I am running my code. can anyone help in this just asking
Exception in thread "main" java.util.FormatFlagsConversionMismatchException: Conversion = s, Flags =
at java.util.Formatter$FormatSpecifier.failMismatch(Formatter.java:4298)
at java.util.Formatter$FormatSpecifier.checkBadFlags(Formatter.java:2997)
at java.util.Formatter$FormatSpecifier.checkGeneral(Formatter.java:2955)
at java.util.Formatter$FormatSpecifier.<init>(Formatter.java:2725)
at java.util.Formatter.parse(Formatter.java:2560)
at java.util.Formatter.format(Formatter.java:2501)
at java.util.Formatter.format(Formatter.java:2455)
at java.lang.String.format(String.java:2940)
at model.Song.toString(Song.java:29)
at java.util.Formatter$FormatSpecifier.printString(Formatter.java:2886)
at java.util.Formatter$FormatSpecifier.print(Formatter.java:2763)
at java.util.Formatter.format(Formatter.java:2520)
at java.io.PrintStream.format(PrintStream.java:970)
at java.io.PrintStream.printf(PrintStream.java:871)
at model.Karaoke.main(Karaoke.java:16)
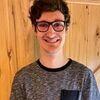
Trent Christofferson
18,846 PointsYour error is at line 16 in Karaoke.java. It is too hard to tell what the problem is without code. When you get errors in the console see if you can find any of your classes that you coded and that is most likely the error your looking for it will tell you the line number. From what I can tell maybe you misused %s or %d or something in a printf?
edard stark
272 Pointsedard stark
272 Pointshey Trent would you please help me with this
e.g. List<Song> mSongs = new ArrayList<>();
for(Song song: msongs)
I know the song is the variable of type Song but how it works, like in the msongs case how it will get all the data from Song. please explain me. Thank You!
Trent Christofferson
18,846 PointsTrent Christofferson
18,846 PointsLoops are truly wonderful! It is very easy to get the data from the song variable. Since the song is of type song it has all of its methods! You can use the methods like so:
What ever you put in the for loop, will run for every stored song inside mSongs. So if you have 10 Songs stored inside mSongs it will run the code 10 times, once every song.
One thing to note is when you are looping through something, let's say mSongs, don't remove a song from the list inside the loop or you will get an error, however, if the code to remove the song from mSongs is not inside the loop you will be just fine. I thought it was worth mentioning as I have made that mistake more than once.