Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial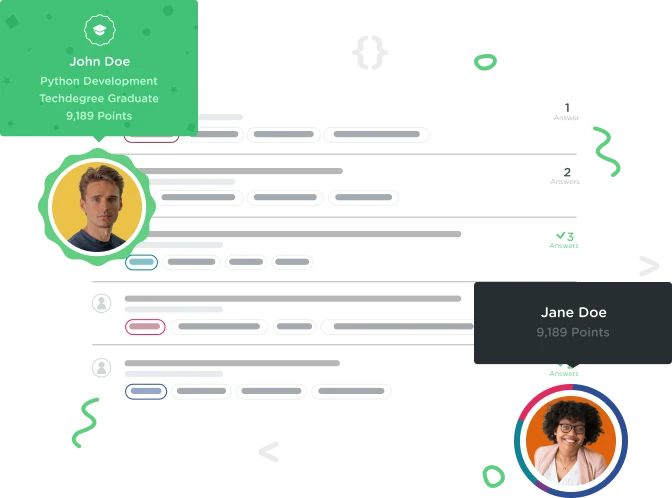
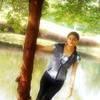
tejaswini vadnal
1,587 Pointshow this works
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$output = $number . $output;
}
}
echo $output;
?>
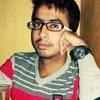
Rangan Roy
1,928 Points1.First $numbers stores the array of 4 elements . 2.$total stores 4(The length of the array). 3.Then in the foreach loop there is a increment of $i and there is check which says that if $i is less than $total , that if block will execute other wise the foreach loop will start again from the next element of the $numbers array .
- At last the output is printed .
- The output will be 3210 .
1 Answer
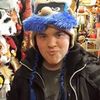
Codin - Codesmite
8,600 Points<?php
$numbers = array(1,2,3,4); // Creates an array called numbers containing 1,2,3,4
$total = count($numbers); // Counts how many values contained in numbers array and assigns to total variable.
$sum = 0; // Sets sum variable to 0
$output = ""; // Sets output variable to an empty string.
$i = 0; // sets i variable to 0.
foreach($numbers as $number) { // Loops each value of numbers array with the current value as number.
$i = $i + 1; // Increments i by 1 by adding 1 to itself and re-declaring i with the incremented value.
if ($i < $total) { // if i is less than total variable (which was set to the amount of values contained in numbers array)
$output = $number . $output; // Set's variable output to the current value with the previous value of output added onto the end using concatenation.
}
}
echo $output; // echos output value of output
?>
This will output "321".
The first loop of the foreach loop will take the value 1 as number from the numbers array, will increment i from 0 to 1, and sets output to "1" (it will only be 1 because output added on the end with concatination is blank".
The second loop of the foreach loop will take the value 2 as number from the numbers array, will increment i from 1 to 2 and sets the output variable to "21" (it concates the current value of number which is "2" with the current value of output which is "1".)
The third loop of the foreach loop will take the value 3 as number from the numbers array, will increment i from 2 to 3, and sets output to "321" (it concates the current value of number which is "3" with the current value of output which is "21")
the fourth loop of the foreach loop will take the value 4 as number from the numbers array, will increment i from 3 to 4, but doesn't change the output as the if statement returns false, as the current value of i is 4 it is not less than the value of total, as total is the value of 4 (a count of the amount of values in the numbers array).
The foreach loop will end as it is now returning false and the last line will echo the current value of output variable which is "321"
Konrad Pilch
2,435 PointsKonrad Pilch
2,435 PointsDo you know how the foreach loop works? You could re-watch the video.