Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial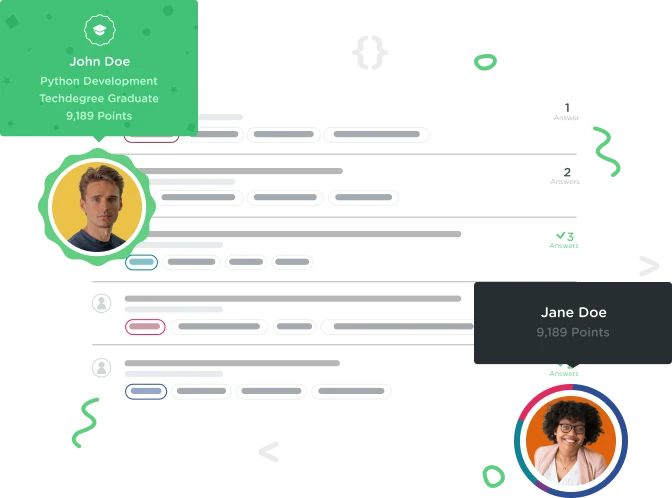

tomasliashuk
8,014 PointsHow those two module patterns are different in practical use?
1 way:
var loginModule = (function(){
var moduleObject = {};
moduleObject.login = function(u,p) {
console.log('Logging IN using "' + u + '" and "' + p + '".');
};
moduleObject.logout = function(u,p) {
console.log('Logging OUT using "' + u + '" and "' + p + '".')
};
return moduleObject;
}());
loginModule.login("myusername", "mypassword");
2 way:
function User() {
var username, password;
function doLogin(u, p) {
username = u;
password = p;
}
var publicAPI = {
login: doLogin
};
return publicAPI;
}
var firstUser = User();
firstUser.login("myusername", "mypassword");

tomasliashuk
8,014 PointsJesus Mendoza , "Btw instead of creating a new object variable, you can return the object directly from the function"
When you say that, are you referring to the first pattern and this object: var moduleObject = {};? If so, how would I return this object directly from the anonymous function, if first I add methods on it?
Can you elaborate, please?
1 Answer

tomasliashuk
8,014 PointsI am going to answer my own question, just in case some one wonders as well. So, there is actually a difference in terms of how and why you would use those patterns.
Take a close look at the first one - it is a singleton module which only has one instance.
The body of a module itself is wrapped into IIFE (immediately-invoked function expression). This basically means that the function will be immediately executed. In this sense the body of a module is once executed and assigned to a variable named "loginModule". What just happened is that we invoked our module from the beginning.
"loginModule" is our single module instance identifier.
Now compare the first one to the second one. Second module is not invoked from the beginning and we use
var firstUser = User();
to invoke the module. The assumption behind this pattern is that you would need to invoke module multiple times.
If someone can add on it I would really appreciate. @hstn
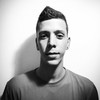
Jesus Mendoza
23,288 PointsYou might be right I didnt notice at first. Thanks for pointing it out
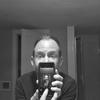
Jason Gresalfi
2,269 PointsThis is a pretty old thread but I'll add my two cents anyway. I agree with @tomasliashuk that the first pattern is a Singleton. The second pattern, I believe is a prototype in that it describes an object (itself) and then self-replicates to create new instances.
Jesus Mendoza
23,288 PointsJesus Mendoza
23,288 PointsI'd say there is no real difference because you can accomplish the same with both but the first one is easier to read.
Btw instead of creating a new object variable, you can return the object directly from the function