Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial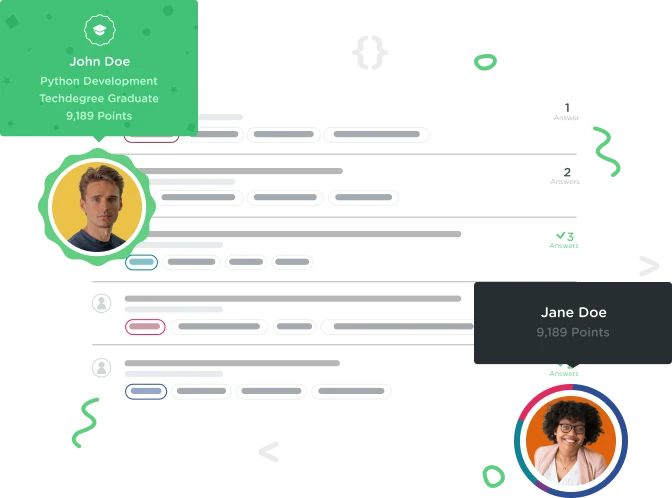

rishavatreya
4,945 PointsHow to access data from blogger( JSON )?
//1 // TableViewController.m // BlogReader
import "TableViewController.h"
@interface TableViewController ()
@end
@implementation TableViewController
(id)initWithStyle:(UITableViewStyle)style { self = [super initWithStyle:style]; if (self) { // Custom initialization } return self; }
-
(void)viewDidLoad { [super viewDidLoad];
NSURL *blogURL = [NSURL URLWithString:@"http://himalayanfood.blogspot.com/feeds/posts/default?alt=json"];
NSData *jsonData = [NSData dataWithContentsOfURL:blogURL];
NSError *error = nil;
NSDictionary *dataDictionary = [NSJSONSerialization JSONObjectWithData:jsonData options:0 error:&error]; NSLog(@"%@",dataDictionary);
self.blogPosts = [dataDictionary objectForKey:@"entry"]; }
(void)didReceiveMemoryWarning { [super didReceiveMemoryWarning]; // Dispose of any resources that can be recreated. }
pragma mark - Table view data source
(NSInteger)numberOfSectionsInTableView:(UITableView *)tableView { // Return the number of sections. return 1; }
(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { // Return the number of rows in the section. return [self.blogPosts count]; }
-
(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString *CellIdentifier = @"Cell"; UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:CellIdentifier forIndexPath:indexPath];
NSDictionary *blogPost = [self.blogPosts objectAtIndex:indexPath.row];
cell.textLabel.text = [blogPost valueForKey:@"type"]; // <------What goes here???? cell.detailTextLabel.text = [blogPost valueForKey:@"$t"];//<------What goes here???? return cell; }
/* // Override to support conditional editing of the table view.
- (BOOL)tableView:(UITableView *)tableView canEditRowAtIndexPath:(NSIndexPath *)indexPath { // Return NO if you do not want the specified item to be editable. return YES; } */
/* // Override to support editing the table view.
- (void)tableView:(UITableView *)tableView commitEditingStyle:(UITableViewCellEditingStyle)editingStyle forRowAtIndexPath:(NSIndexPath *)indexPath { if (editingStyle == UITableViewCellEditingStyleDelete) { // Delete the row from the data source [tableView deleteRowsAtIndexPaths:@[indexPath] withRowAnimation:UITableViewRowAnimationFade]; } else if (editingStyle == UITableViewCellEditingStyleInsert) { // Create a new instance of the appropriate class, insert it into the array, and add a new row to the table view } } */
/* // Override to support rearranging the table view.
- (void)tableView:(UITableView *)tableView moveRowAtIndexPath:(NSIndexPath *)fromIndexPath toIndexPath:(NSIndexPath *)toIndexPath { } */
/* // Override to support conditional rearranging of the table view.
- (BOOL)tableView:(UITableView *)tableView canMoveRowAtIndexPath:(NSIndexPath *)indexPath { // Return NO if you do not want the item to be re-orderable. return YES; } */
pragma mark - Table view delegate
- (void)tableView:(UITableView )tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { // Navigation logic may go here. Create and push another view controller. / <#DetailViewController#> *detailViewController = [[<#DetailViewController#> alloc] initWithNibName:@"<#Nib name#>" bundle:nil]; // ... // Pass the selected object to the new view controller. [self.navigationController pushViewController:detailViewController animated:YES]; */ }
@end
2 Answers
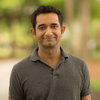
Amit Bijlani
Treehouse Guest TeacherThe way the data is structured you have a dictionary inside a dictionary. Feed (dictionary) -> Entry (array of dictionaries). To access nested structures you would have to use the method valueForKeyPath
. In the example above you could do the following:
self.blogPosts = [dataDictionary valueForKeyPath:@"feed.entry"];
Notice how the two keys feed
and entry
are separated by a period. It's the easiest way to traverse nested nodes and get access to a leaf node.

rishavatreya
4,945 Pointsplease see the comment line '//<-----what goes here?' json linke is here. http://himalayanfood.blogspot.com/feeds/posts/default?alt=json which displays the blog title slightly different way than on the http://blog.teamtreehouse.com/api/get_recent_summary/

rishavatreya
4,945 Pointsrishavatreya
4,945 PointsThank you very much!