Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial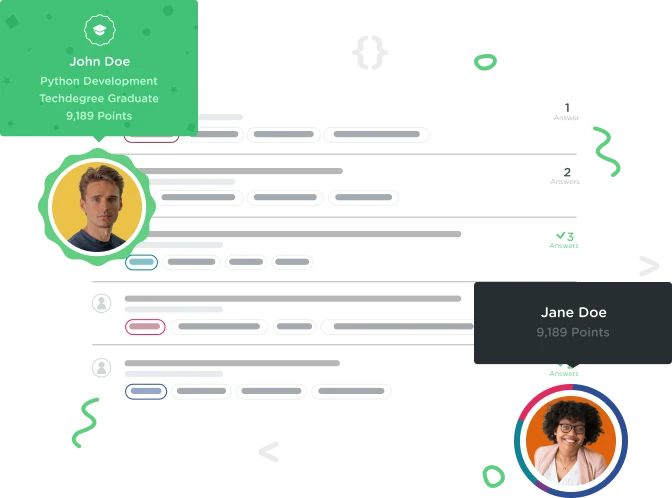

Bojan Hinic
1,477 PointsHow to access named parameter when call function?
Hi everyone,
After calling function I'm trying to access parameter with (number) and (by) interpolation but returns error (Use of unresolved identifier 'number' and 'by').
How can I access these values?
func isDivisible(#number: Int, #by: Int) -> Bool?{
if number % by == 0{
return true
}else{
return nil
}
}
if let test = isDivisible(number: 65,by: 5){
println("\(number) is divisible \(by).")
} else{
println("Not divisible.")
}
2 Answers
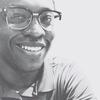
Ricardo Hill-Henry
38,442 PointsI don't believe you had to do print them out like so. However, if you're just trying to experiment, I'll have a go.
First, you can't access a parameter's value because they don't actually store a value. Parameters give you the ability to take an inputted value, and store it in a variable or constant, or manipulate a value within a function. If you want to access the value that was input into the function, you'll need some way of storing said value(s). If you want to access the values inside the function outside the function's scope you'll need to use global variables (variables declared at the top of the program, or in this case just before the function's declaration).
var numberVar = 0
var byVar = 0
func isDivisible(#number: Int, #by: Int) -> Bool?{
numberVar = number
byVar = by
if number % by == 0{
return true
}else{
return nil
}
}
if let test = isDivisible(number: 65, by: 5){
println("\(numberVar) is divisible \(byVar).")
} else{
println("Not divisible.")
}
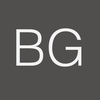
Burak Gür
3,747 Pointsfunc isDivisible(#number: Int, #by: Int) -> Bool?{
if number % by == 0{
return true
}else{
return nil
}
}
var number1: Int = 65
var number2: Int = 5
if let test = isDivisible(number: number1, by: number2){
println("\(number1) is divisible \(number2).")
} else{
println("Not divisible.")
}

Bojan Hinic
1,477 PointsThank you for answering. I appreciate your help.
Bojan Hinic
1,477 PointsBojan Hinic
1,477 PointsRicardo your explanation was really helpful. Thank you for your time.