Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial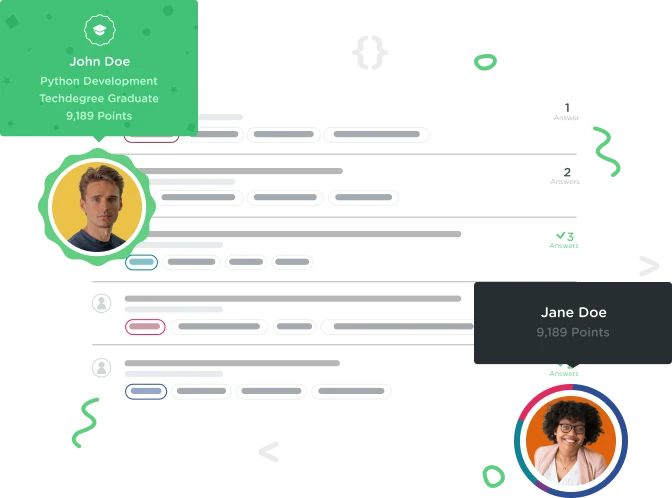

German Espitia
4,578 PointsHow to access properties of another class?
I'm trying to access and modify a property of class A in class B
ClassB.h
@property (strong, nonatomic) ClassA * pointerToClassA;
ClassB.m
[pointerToClassA addObject:someObject];
What am I doing wrong?
3 Answers

Stone Preston
42,016 Pointsif ClassA has an NSArray property called someArray and you create an instance of Class A called objectA in some other class, you would access that object's someArray property and and an object to it like this:
[objectA.someArray addObject:someObject];
to get what you are doing currently to work, you would need to do something like
[pointerToClassA.theNameOfTheArrayProperty addObject:someObject];
also, your object names are a bit misleading (ClassA *pointerToClassA). when you create an object, it doesnt point to the class it is. it points to the memory address where the object is located. A more fitting name would probably be something like ClassA objectOfTypeClassA or something

German Espitia
4,578 Pointsdouble answer

Stone Preston
42,016 Pointsif you are using storyboards what you typically do to pass data between view controllers is use prepare for segue. You need to add an NSMutableArray property to your AddHabitsViewController to store the data from the array in HabitsViewController. Then in prepareForSegue you can pass the data like so:
//in HabitsViewController
- (void)prepareForSegue:(UIStoryboardSegue *)segue sender:(id)sender {
if([segue.identifier isEqualToString:@"showAddHabit"]) {
AddHabitViewController *viewController = (AddHabitViewController *)segue.destinationViewController;
viewController.habits = [NSMutableArray arrayWithArray:self.habits];
}
}
then when you segue to the viewController, it will have the data from the HabitsViewController in its own array.

German Espitia
4,578 Pointsso when I edit that array in HabitsViewController, how do I pass it back? sorry for asking so much.

Stone Preston
42,016 Pointsare you saving anything to a backend? if so then you would just save it to the backend and then your habitsViewController queries the backend when it appears to get any new data that was added. if you need to pass data around back and forth to multiple view controllers it might be better to implement a singleton class as a data model

German Espitia
4,578 PointsYup that's exactly what I did as I mentioned in the last edit of the last answer. I just wasn't sure if that was the best practice or better to send info back and forth through viewcontrollers.
Thank you!
German Espitia
4,578 PointsGerman Espitia
4,578 PointsSorry, I had my code just as you said, which works to access the property.
The real problem I have is that for some reason, the mutableArray in class A shows as empty in classB. So the question isn't how to access it, but why does it show empty in classB?
Stone Preston
42,016 PointsStone Preston
42,016 Pointslets say you have a class called classA that has an NSArray property called someArray. if I were to create an object of ClassA named object1 I could set that objects array property like this
[object1.someArray = @[@"some stuff", @"some more stuff"]
. then I could create another object of classA called object2 and access its array property like this[object1.someArray = @[@"some other stuff", @"stuff"]
.the 2 objects have 2 different array properties. thats most likely whats happening in your case. you have 2 different objects being created.
German Espitia
4,578 PointsGerman Espitia
4,578 PointsSo how do I make an instance of a property of another class? If I'm using the code below to access
arrayFromClassA
of ClassA in ClassB, why do I get an empty version of the array when in class A it has many objects?ClassB.h
@property (strong, nonatomic) ClassA * pointerToClassA;
ClassB.m
[pointerToClassA.arrayFromClassA count];
Stone Preston
42,016 PointsStone Preston
42,016 Pointscan you explain less abstractly what youd like to accomplish? do you have a view controller you want to pass information to for example?
German Espitia
4,578 PointsGerman Espitia
4,578 PointsI have a class named HabitsViewController that has a
NSMutableArray
that holds the habit objects of a user. When clicking the add button, they get taken to another view called AddHabitViewController where they input some information. From this info, I am trying to add an object to theNSMutableArray
of HabitsViewController.Hope that explains it well. I appreciate all your help by the way! I've learned a lot already from you.
EDIT: I solved the problem by doing it over Parse.com to reload the data in the first view controller (that lists all the habits). I think it might be slower like this but it gets the job done :)