Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial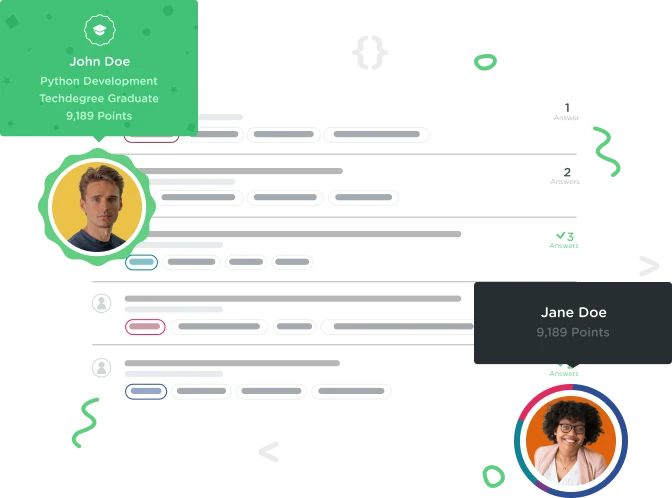
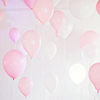
Dylan Cairns
11,191 PointsHow to access strings.xml from the new classes?
I'm following along with the new first lesson: building a simple android app and I wanted to use the string.xml resource instead of hardcoding the facts and hexadecimals. But I cannot access it from the new classes I make FactBook and ColorWheel.java
Trying this inside of FactBook.java
public String[] mFacts = Context.getResources().getStringArray(R.array.array_of_facts);
I get an error "non-static method 'getResources() cannot be referenced from a static context"
.
These are my arrays in the .xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<string name="app_name">Fun Facts</string>
<string name="hello_world">Hello world!</string>
<string name="action_settings">Settings</string>
<string name="did_you_know">Did you konw?</string>
<string name="fact_ants">Ants stretch when they wake up in the morning.</string>
<string name="button_show_another_fact">Show Another Fun Fact</string>
<string-array name="array_of_facts">
<item> meow 1 </item>
<item> meow 2</item>
<item> meow 3</item>
<item> meow 4</item>
<item> meow 5</item>
</string-array>
<string-array name="array_of_colors">
<item> #39add1 </item>
<item> #3079ab</item>
<item> #838cc7</item>
<item> #53bbb4</item>
<item> #e15258</item>
</string-array>
</resources>
I've been searching everywhere but don't understand how to get the context or whatever I need for these new classes in order to access the resources
For reference this is my FactBook code:
package com.endofdaze.funfacts;
import android.content.Context;
import java.util.Random;
public class FactBook {
public String[] mFacts = Context.getResources().getStringArray(R.array.array_of_facts);
public String mFact = null;
public String getFact() {
// Randomly select a fact
Random randomGenerator = new Random(); // construct new RNG
int randomNumber = randomGenerator.nextInt(mFacts.length);
mFact = mFacts[randomNumber];
return mFact;
}
}
2 Answers
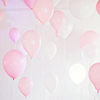
Dylan Cairns
11,191 PointsThrough a lot of trial and error I think I got this to work. I had to extend ContextWrapper to the ColorWheel and FactBook classes and give them the context of the main activity
package com.endofdaze.funfacts;
import android.content.Context;
import android.content.ContextWrapper;
import android.graphics.Color;
import java.util.Random;
public class ColorWheel extends ContextWrapper {
private String[] mColors;
private String mColor = "";
public ColorWheel(Context base) {
super(base);
mColors = getResources().getStringArray(R.array.array_of_colors);
mColor = "";
}
then I had to create the instances of these classes inside of the OnCreate method as "final" rather than at the top of the class itself
package com.endofdaze.funfacts;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class FunFactsActivity extends Activity {
public static final String TAG = FunFactsActivity.class.getSimpleName();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts);
// declare view variables & assign the views from layout file.
final TextView factLabel = (TextView)findViewById(R.id.factTextView);
final Button showFactButton = (Button)findViewById(R.id.showFactButton);
final RelativeLayout layout = (RelativeLayout)findViewById(R.id.relativeLayout);
final FactBook mFactBook = (new FactBook(this));
final ColorWheel mColorWheel = (new ColorWheel(this));
if anyone else is interested in getting rid of their hardcoded Strings then I hope this helps you out too. It took a few hours to figure it out, as simple as it seems!

Barry May
3,963 PointsThanks for this. Much simpler approach than any others I found online!
Klemen Pravdic
10,150 PointsKlemen Pravdic
10,150 PointsNice
Trainer Workout
22,341 PointsTrainer Workout
22,341 PointsHow did you access the color value from the xml in your Java code ? I ask this because I can access the color reference (@color/android) but I don't know how to use it.